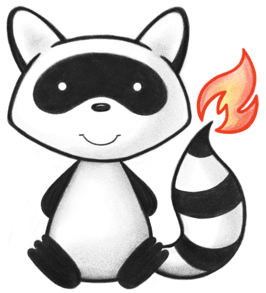
001/*- 002 * #%L 003 * HAPI FHIR JPA Server - International Patient Summary (IPS) 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.ips.api; 021 022import org.hl7.fhir.instance.model.api.IBaseResource; 023import org.hl7.fhir.instance.model.api.IIdType; 024 025public class IpsContext { 026 027 private final IBaseResource mySubject; 028 private final IIdType mySubjectId; 029 030 /** 031 * Constructor 032 * 033 * @param theSubject The subject Patient resource for the IPS being generated 034 * @param theSubjectId The original ID for {@literal theSubject}, which may not match the current ID if {@link IIpsGenerationStrategy#massageResourceId(IpsContext, IBaseResource)} has modified it 035 */ 036 public IpsContext(IBaseResource theSubject, IIdType theSubjectId) { 037 mySubject = theSubject; 038 mySubjectId = theSubjectId; 039 } 040 041 /** 042 * Returns the subject Patient resource for the IPS being generated. Note that 043 * the {@literal Resource.id} value may not match the ID of the resource stored in the 044 * repository if {@link IIpsGenerationStrategy#massageResourceId(IpsContext, IBaseResource)} has 045 * returned a different ID. Use {@link #getSubjectId()} if you want the originally stored ID. 046 * 047 * @see #getSubjectId() for the originally stored ID. 048 */ 049 public IBaseResource getSubject() { 050 return mySubject; 051 } 052 053 /** 054 * Returns the ID of the subject for the given IPS. This value should match the 055 * ID which was originally fetched from the repository. 056 */ 057 public IIdType getSubjectId() { 058 return mySubjectId; 059 } 060 061 public <T extends IBaseResource> IpsSectionContext<T> newSectionContext( 062 Section theSection, Class<T> theResourceType) { 063 return new IpsSectionContext<>(mySubject, mySubjectId, theSection, theResourceType); 064 } 065}