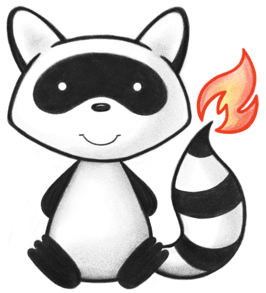
001/*- 002 * #%L 003 * HAPI FHIR JPA Server - International Patient Summary (IPS) 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.ips.jpa.section; 021 022import ca.uhn.fhir.jpa.ips.api.IpsSectionContext; 023import ca.uhn.fhir.jpa.ips.jpa.JpaSectionSearchStrategy; 024import ca.uhn.fhir.jpa.searchparam.SearchParameterMap; 025import ca.uhn.fhir.rest.param.TokenOrListParam; 026import ca.uhn.fhir.rest.param.TokenParam; 027import jakarta.annotation.Nonnull; 028import org.hl7.fhir.r4.model.Observation; 029 030import static ca.uhn.fhir.jpa.term.api.ITermLoaderSvc.LOINC_URI; 031 032public class PregnancyJpaSectionSearchStrategy extends JpaSectionSearchStrategy<Observation> { 033 034 public static final String LOINC_CODE_PREGNANCY_STATUS = "82810-3"; 035 public static final String LOINC_CODE_NUMBER_BIRTHS_LIVE = "11636-8"; 036 public static final String LOINC_CODE_NUMBER_BIRTHS_PRETERM = "11637-6"; 037 public static final String LOINC_CODE_NUMBER_BIRTHS_STILL_LIVING = "11638-4"; 038 public static final String LOINC_CODE_NUMBER_BIRTHS_TERM = "11639-2"; 039 public static final String LOINC_CODE_NUMBER_BIRTHS_TOTAL = "11640-0"; 040 public static final String LOINC_CODE_NUMBER_ABORTIONS = "11612-9"; 041 public static final String LOINC_CODE_NUMBER_ABORTIONS_INDUCED = "11613-7"; 042 public static final String LOINC_CODE_NUMBER_ABORTIONS_SPONTANEOUS = "11614-5"; 043 public static final String LOINC_CODE_NUMBER_ECTOPIC_PREGNANCY = "33065-4"; 044 045 @Override 046 public void massageResourceSearch( 047 @Nonnull IpsSectionContext<Observation> theIpsSectionContext, 048 @Nonnull SearchParameterMap theSearchParameterMap) { 049 theSearchParameterMap.add( 050 Observation.SP_CODE, 051 new TokenOrListParam() 052 .addOr(new TokenParam(LOINC_URI, LOINC_CODE_PREGNANCY_STATUS)) 053 .addOr(new TokenParam(LOINC_URI, LOINC_CODE_NUMBER_BIRTHS_LIVE)) 054 .addOr(new TokenParam(LOINC_URI, LOINC_CODE_NUMBER_BIRTHS_PRETERM)) 055 .addOr(new TokenParam(LOINC_URI, LOINC_CODE_NUMBER_BIRTHS_STILL_LIVING)) 056 .addOr(new TokenParam(LOINC_URI, LOINC_CODE_NUMBER_BIRTHS_TERM)) 057 .addOr(new TokenParam(LOINC_URI, LOINC_CODE_NUMBER_BIRTHS_TOTAL)) 058 .addOr(new TokenParam(LOINC_URI, LOINC_CODE_NUMBER_ABORTIONS)) 059 .addOr(new TokenParam(LOINC_URI, LOINC_CODE_NUMBER_ABORTIONS_INDUCED)) 060 .addOr(new TokenParam(LOINC_URI, LOINC_CODE_NUMBER_ABORTIONS_SPONTANEOUS)) 061 .addOr(new TokenParam(LOINC_URI, LOINC_CODE_NUMBER_ECTOPIC_PREGNANCY))); 062 } 063 064 @SuppressWarnings("RedundantIfStatement") 065 @Override 066 public boolean shouldInclude( 067 @Nonnull IpsSectionContext<Observation> theIpsSectionContext, @Nonnull Observation theCandidate) { 068 // code filtering not yet applied 069 if (theCandidate.getStatus() == Observation.ObservationStatus.PRELIMINARY) { 070 return false; 071 } 072 073 return true; 074 } 075}