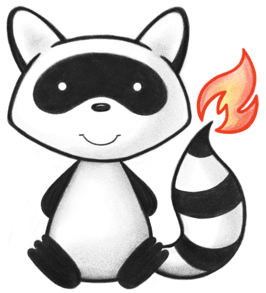
001/*- 002 * #%L 003 * HAPI FHIR JPA Server - International Patient Summary (IPS) 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.ips.provider; 021 022import ca.uhn.fhir.jpa.ips.generator.IIpsGeneratorSvc; 023import ca.uhn.fhir.jpa.model.util.JpaConstants; 024import ca.uhn.fhir.model.api.annotation.Description; 025import ca.uhn.fhir.model.valueset.BundleTypeEnum; 026import ca.uhn.fhir.rest.annotation.IdParam; 027import ca.uhn.fhir.rest.annotation.Operation; 028import ca.uhn.fhir.rest.annotation.OperationParam; 029import ca.uhn.fhir.rest.api.server.RequestDetails; 030import ca.uhn.fhir.rest.param.TokenParam; 031import ca.uhn.fhir.util.FhirTerser; 032import ca.uhn.fhir.util.ValidateUtil; 033import jakarta.annotation.Nonnull; 034import org.hl7.fhir.instance.model.api.IBase; 035import org.hl7.fhir.instance.model.api.IBaseBundle; 036import org.hl7.fhir.instance.model.api.IIdType; 037import org.hl7.fhir.instance.model.api.IPrimitiveType; 038import org.thymeleaf.util.Validate; 039 040public class IpsOperationProvider { 041 042 private final IIpsGeneratorSvc myIpsGeneratorSvc; 043 044 /** 045 * Constructor 046 */ 047 public IpsOperationProvider(@Nonnull IIpsGeneratorSvc theIpsGeneratorSvc) { 048 Validate.notNull(theIpsGeneratorSvc, "theIpsGeneratorSvc must not be null"); 049 myIpsGeneratorSvc = theIpsGeneratorSvc; 050 } 051 052 /** 053 * Patient/123/$summary 054 * <p> 055 * Note that not all parameters from the official specification are yet supported. See 056 * <a href="http://build.fhir.org/ig/HL7/fhir-ips/OperationDefinition-summary.html>http://build.fhir.org/ig/HL7/fhir-ips/OperationDefinition-summary.html</a> 057 */ 058 @Operation( 059 name = JpaConstants.OPERATION_SUMMARY, 060 idempotent = true, 061 bundleType = BundleTypeEnum.DOCUMENT, 062 typeName = "Patient", 063 canonicalUrl = JpaConstants.SUMMARY_OPERATION_URL) 064 public IBaseBundle patientInstanceSummary( 065 @IdParam IIdType thePatientId, 066 @OperationParam(name = "profile", min = 0, typeName = "uri") IPrimitiveType<String> theProfile, 067 RequestDetails theRequestDetails) { 068 String profile = theProfile != null ? theProfile.getValueAsString() : null; 069 return myIpsGeneratorSvc.generateIps(theRequestDetails, thePatientId, profile); 070 } 071 072 /** 073 * /Patient/$summary?identifier=foo|bar 074 * <p> 075 * Note that not all parameters from the official specification are yet supported. See 076 * <a href="http://build.fhir.org/ig/HL7/fhir-ips/OperationDefinition-summary.html>http://build.fhir.org/ig/HL7/fhir-ips/OperationDefinition-summary.html</a> 077 */ 078 @Operation( 079 name = JpaConstants.OPERATION_SUMMARY, 080 idempotent = true, 081 bundleType = BundleTypeEnum.DOCUMENT, 082 typeName = "Patient", 083 canonicalUrl = JpaConstants.SUMMARY_OPERATION_URL) 084 public IBaseBundle patientTypeSummary( 085 @OperationParam(name = "profile", min = 0, typeName = "uri") IPrimitiveType<String> theProfile, 086 @Description( 087 shortDefinition = 088 "When the logical id of the patient is not used, servers MAY choose to support patient selection based on provided identifier") 089 @OperationParam(name = "identifier", min = 1, max = 1, typeName = "Identifier") 090 IBase thePatientIdentifier, 091 RequestDetails theRequestDetails) { 092 String profile = theProfile != null ? theProfile.getValueAsString() : null; 093 094 ValidateUtil.isTrueOrThrowInvalidRequest(thePatientIdentifier != null, "No ID or identifier supplied"); 095 096 FhirTerser terser = theRequestDetails.getFhirContext().newTerser(); 097 String system = terser.getSinglePrimitiveValueOrNull(thePatientIdentifier, "system"); 098 String value = terser.getSinglePrimitiveValueOrNull(thePatientIdentifier, "value"); 099 return myIpsGeneratorSvc.generateIps(theRequestDetails, new TokenParam(system, value), profile); 100 } 101}