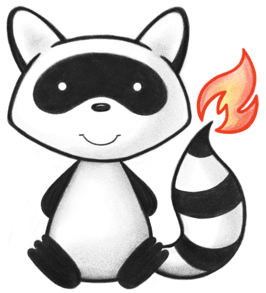
001/*- 002 * #%L 003 * HAPI FHIR JPA Server - International Patient Summary (IPS) 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.ips.strategy; 021 022import ca.uhn.fhir.jpa.ips.api.IIpsGenerationStrategy; 023import ca.uhn.fhir.jpa.ips.api.ISectionResourceSupplier; 024import ca.uhn.fhir.jpa.ips.api.IpsContext; 025import ca.uhn.fhir.jpa.ips.api.Section; 026import com.google.common.collect.Lists; 027import jakarta.annotation.Nonnull; 028import jakarta.annotation.Nullable; 029import org.hl7.fhir.instance.model.api.IBaseResource; 030import org.hl7.fhir.instance.model.api.IIdType; 031import org.hl7.fhir.r4.model.Address; 032import org.hl7.fhir.r4.model.Composition; 033import org.hl7.fhir.r4.model.IdType; 034import org.hl7.fhir.r4.model.Organization; 035import org.thymeleaf.util.Validate; 036 037import java.time.LocalDate; 038import java.time.format.DateTimeFormatter; 039import java.util.ArrayList; 040import java.util.Collections; 041import java.util.HashMap; 042import java.util.List; 043import java.util.Map; 044 045@SuppressWarnings({"HttpUrlsUsage"}) 046public abstract class BaseIpsGenerationStrategy implements IIpsGenerationStrategy { 047 048 public static final String DEFAULT_IPS_NARRATIVES_PROPERTIES = 049 "classpath:ca/uhn/fhir/jpa/ips/narrative/ips-narratives.properties"; 050 private final List<Section> mySections = new ArrayList<>(); 051 private final Map<Section, ISectionResourceSupplier> mySectionToResourceSupplier = new HashMap<>(); 052 053 /** 054 * Constructor 055 */ 056 public BaseIpsGenerationStrategy() { 057 super(); 058 } 059 060 @Override 061 public String getBundleProfile() { 062 return "http://hl7.org/fhir/uv/ips/StructureDefinition/Bundle-uv-ips"; 063 } 064 065 @Nonnull 066 @Override 067 public final List<Section> getSections() { 068 return Collections.unmodifiableList(mySections); 069 } 070 071 @Nonnull 072 @Override 073 public ISectionResourceSupplier getSectionResourceSupplier(@Nonnull Section theSection) { 074 return mySectionToResourceSupplier.get(theSection); 075 } 076 077 /** 078 * This should be called once per section to add a section for inclusion in generated IPS documents. 079 * It should include a {@link Section} which contains static details about the section, and a {@link ISectionResourceSupplier} 080 * which is used to fetch resources for inclusion at runtime. 081 * 082 * @param theSection Contains static details about the section, such as the resource types it can contain, and a title. 083 * @param theSectionResourceSupplier The strategy object which will be used to supply content for this section at runtime. 084 */ 085 public void addSection(Section theSection, ISectionResourceSupplier theSectionResourceSupplier) { 086 Validate.notNull(theSection, "theSection must not be null"); 087 Validate.notNull(theSectionResourceSupplier, "theSectionResourceSupplier must not be null"); 088 Validate.isTrue( 089 !mySectionToResourceSupplier.containsKey(theSection), 090 "A section with the given profile already exists"); 091 092 mySections.add(theSection); 093 mySectionToResourceSupplier.put(theSection, theSectionResourceSupplier); 094 } 095 096 @Override 097 public List<String> getNarrativePropertyFiles() { 098 return Lists.newArrayList(DEFAULT_IPS_NARRATIVES_PROPERTIES); 099 } 100 101 @Override 102 public IBaseResource createAuthor() { 103 Organization organization = new Organization(); 104 organization 105 .setName("eHealthLab - University of Cyprus") 106 .addAddress(new Address() 107 .addLine("1 University Avenue") 108 .setCity("Nicosia") 109 .setPostalCode("2109") 110 .setCountry("CY")) 111 .setId(IdType.newRandomUuid()); 112 return organization; 113 } 114 115 @Override 116 public String createTitle(IpsContext theContext) { 117 return "Patient Summary as of " 118 + DateTimeFormatter.ofPattern("MM/dd/yyyy").format(LocalDate.now()); 119 } 120 121 @Override 122 public String createConfidentiality(IpsContext theIpsContext) { 123 return Composition.DocumentConfidentiality.N.toCode(); 124 } 125 126 @Override 127 public IIdType massageResourceId(@Nullable IpsContext theIpsContext, @Nonnull IBaseResource theResource) { 128 return null; 129 } 130}