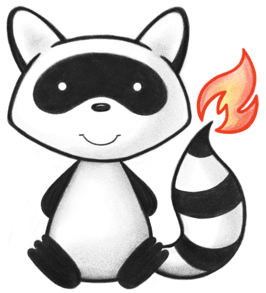
001/*- 002 * #%L 003 * HAPI FHIR JPA Model 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.model.dao; 021 022import ca.uhn.fhir.jpa.model.entity.PartitionablePartitionId; 023import ca.uhn.hapi.fhir.sql.hibernatesvc.PartitionedIdProperty; 024import jakarta.persistence.Column; 025import jakarta.persistence.Embeddable; 026 027import java.io.Serializable; 028import java.util.List; 029import java.util.Objects; 030import java.util.stream.Collectors; 031 032/** 033 * This is a version of {@link JpaPid} which can be used as a FK, since it doesn't include 034 * the {@literal @Id} or related annotations. 035 */ 036@Embeddable 037public class JpaPidFk implements Serializable { 038 039 @Column(name = "RES_ID", nullable = false) 040 private Long myId; 041 042 @PartitionedIdProperty 043 @Column(name = PartitionablePartitionId.PARTITION_ID, nullable = false) 044 private Integer myPartitionIdValue; 045 046 /** 047 * Note that equals and hashCode for this object only consider the ID and Partition ID because 048 * this class gets used as cache keys 049 */ 050 @Override 051 public boolean equals(Object theO) { 052 if (this == theO) { 053 return true; 054 } 055 if (!(theO instanceof JpaPidFk)) { 056 return false; 057 } 058 JpaPidFk jpaPid = (JpaPidFk) theO; 059 return Objects.equals(myId, jpaPid.myId) && Objects.equals(myPartitionIdValue, jpaPid.myPartitionIdValue); 060 } 061 062 /** 063 * Note that equals and hashCode for this object only consider the ID and Partition ID because 064 * this class gets used as cache keys 065 */ 066 @Override 067 public int hashCode() { 068 return Objects.hash(myId, myPartitionIdValue); 069 } 070 071 public JpaPid toJpaPid() { 072 return JpaPid.fromId(myId, myPartitionIdValue); 073 } 074 075 public void setId(Long theId) { 076 myId = theId; 077 } 078 079 public void setPartitionId(Integer thePartitionId) { 080 myPartitionIdValue = thePartitionId; 081 } 082 083 public static List<JpaPidFk> fromPids(List<JpaPid> thePids) { 084 return thePids.stream().map(JpaPidFk::fromPid).collect(Collectors.toList()); 085 } 086 087 public static JpaPidFk fromPid(JpaPid thePid) { 088 JpaPidFk retVal = new JpaPidFk(); 089 retVal.setId(thePid.getId()); 090 retVal.setPartitionId(thePid.getPartitionId()); 091 return retVal; 092 } 093 094 public static JpaPidFk fromId(Long theId, Integer thePartitionId) { 095 JpaPidFk retVal = new JpaPidFk(); 096 retVal.setId(theId); 097 retVal.setPartitionId(thePartitionId); 098 return retVal; 099 } 100 101 public static JpaPidFk fromId(Long theId) { 102 return fromId(theId, null); 103 } 104}