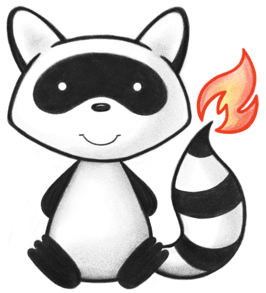
001/* 002 * #%L 003 * HAPI FHIR JPA Model 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.model.entity; 021 022import ca.uhn.fhir.context.FhirVersionEnum; 023import ca.uhn.fhir.jpa.model.cross.IBasePersistedResource; 024import ca.uhn.fhir.jpa.model.dao.JpaPid; 025import ca.uhn.fhir.model.primitive.InstantDt; 026import jakarta.persistence.Column; 027import jakarta.persistence.EnumType; 028import jakarta.persistence.Enumerated; 029import jakarta.persistence.MappedSuperclass; 030import jakarta.persistence.Temporal; 031import jakarta.persistence.TemporalType; 032import org.hibernate.annotations.OptimisticLock; 033 034import java.util.Collection; 035import java.util.Date; 036 037@MappedSuperclass 038public abstract class BaseHasResource extends BasePartitionable 039 implements IBaseResourceEntity, IBasePersistedResource<JpaPid> { 040 041 public static final String RES_PUBLISHED = "RES_PUBLISHED"; 042 public static final String RES_UPDATED = "RES_UPDATED"; 043 044 @Column(name = "RES_DELETED_AT", nullable = true) 045 @Temporal(TemporalType.TIMESTAMP) 046 private Date myDeleted; 047 048 @Column(name = "RES_VERSION", nullable = true, length = 7) 049 @Enumerated(EnumType.STRING) 050 @OptimisticLock(excluded = true) 051 private FhirVersionEnum myFhirVersion; 052 053 @Column(name = "HAS_TAGS", nullable = false) 054 @OptimisticLock(excluded = true) 055 private boolean myHasTags; 056 057 @Temporal(TemporalType.TIMESTAMP) 058 @Column(name = RES_PUBLISHED, nullable = false) 059 @OptimisticLock(excluded = true) 060 private Date myPublished; 061 062 @Temporal(TemporalType.TIMESTAMP) 063 @Column(name = RES_UPDATED, nullable = false) 064 @OptimisticLock(excluded = true) 065 private Date myUpdated; 066 067 public abstract BaseTag addTag(TagDefinition theDef); 068 069 @Override 070 public Date getDeleted() { 071 return cloneDate(myDeleted); 072 } 073 074 @Override 075 public FhirVersionEnum getFhirVersion() { 076 return myFhirVersion; 077 } 078 079 public void setFhirVersion(FhirVersionEnum theFhirVersion) { 080 myFhirVersion = theFhirVersion; 081 } 082 083 public void setDeleted(Date theDate) { 084 myDeleted = theDate; 085 } 086 087 @Override 088 public InstantDt getPublished() { 089 if (myPublished != null) { 090 return new InstantDt(getPublishedDate()); 091 } else { 092 return null; 093 } 094 } 095 096 public Date getPublishedDate() { 097 return cloneDate(myPublished); 098 } 099 100 public void setPublished(Date thePublished) { 101 myPublished = thePublished; 102 } 103 104 public void setPublished(InstantDt thePublished) { 105 myPublished = thePublished.getValue(); 106 } 107 108 public abstract Collection<? extends BaseTag> getTags(); 109 110 @Override 111 public InstantDt getUpdated() { 112 return new InstantDt(getUpdatedDate()); 113 } 114 115 @Override 116 public Date getUpdatedDate() { 117 return cloneDate(myUpdated); 118 } 119 120 public void setUpdated(Date theUpdated) { 121 myUpdated = theUpdated; 122 } 123 124 @Override 125 public boolean isHasTags() { 126 return myHasTags; 127 } 128 129 public void setHasTags(boolean theHasTags) { 130 myHasTags = theHasTags; 131 } 132 133 static Date cloneDate(Date theDate) { 134 Date retVal = theDate; 135 if (retVal != null) { 136 retVal = new Date(retVal.getTime()); 137 } 138 return retVal; 139 } 140}