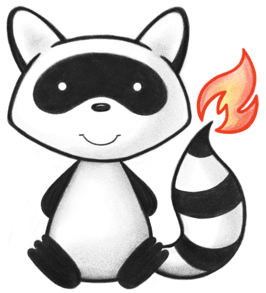
001/*- 002 * #%L 003 * HAPI FHIR JPA Model 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.model.entity; 021 022import jakarta.persistence.MappedSuperclass; 023import org.apache.commons.lang3.ObjectUtils; 024 025import java.io.Serializable; 026 027@MappedSuperclass 028public abstract class BaseResourceIndex extends BasePartitionable implements Serializable { 029 030 public abstract Long getId(); 031 032 public abstract void setId(Long theId); 033 034 public abstract void calculateHashes(); 035 036 public abstract void clearHashes(); 037 038 @Override 039 public void setPartitionId(PartitionablePartitionId thePartitionId) { 040 if (ObjectUtils.notEqual(getPartitionId(), thePartitionId)) { 041 super.setPartitionId(thePartitionId); 042 clearHashes(); 043 } 044 } 045 046 /** 047 * Subclasses must implement 048 */ 049 @Override 050 public abstract int hashCode(); 051 052 /** 053 * Subclasses must implement 054 */ 055 @Override 056 public abstract boolean equals(Object obj); 057 058 public abstract <T extends BaseResourceIndex> void copyMutableValuesFrom(T theSource); 059 060 /** 061 * This is called when reindexing a resource on the previously existing index rows. This method 062 * should set zero/0 values for the hashes, in order to avoid any calculating hashes on existing 063 * rows failing. This is important only in cases where hashes are not present on the existing rows, 064 * which would only be the case if new hash columns have been added. 065 */ 066 public void setPlaceholderHashesIfMissing() { 067 // nothing by default 068 } 069 070 public abstract void setResourceId(Long theId); 071}