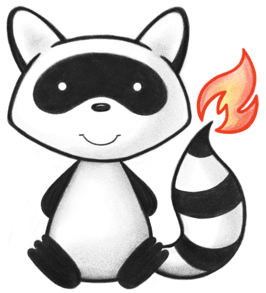
001/* 002 * #%L 003 * HAPI FHIR JPA Model 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.model.entity; 021 022import ca.uhn.fhir.interceptor.model.RequestPartitionId; 023import ca.uhn.fhir.jpa.model.config.PartitionSettings; 024import jakarta.persistence.Column; 025import jakarta.persistence.MappedSuperclass; 026import org.apache.commons.lang3.builder.HashCodeBuilder; 027import org.hibernate.search.mapper.pojo.mapping.definition.annotation.FullTextField; 028 029import static ca.uhn.fhir.jpa.model.util.SearchParamHash.hashSearchParam; 030 031@MappedSuperclass 032public abstract class BaseResourceIndexedSearchParamQuantity extends BaseResourceIndexedSearchParam { 033 034 private static final int MAX_LENGTH = 200; 035 036 private static final long serialVersionUID = 1L; 037 038 @Column(name = "SP_SYSTEM", nullable = true, length = MAX_LENGTH) 039 @FullTextField 040 public String mySystem; 041 042 @Column(name = "SP_UNITS", nullable = true, length = MAX_LENGTH) 043 @FullTextField 044 public String myUnits; 045 046 /** 047 * @since 3.5.0 - At some point this should be made not-null 048 */ 049 @Column(name = "HASH_IDENTITY_AND_UNITS", nullable = true) 050 private Long myHashIdentityAndUnits; 051 /** 052 * @since 3.5.0 - At some point this should be made not-null 053 */ 054 @Column(name = "HASH_IDENTITY_SYS_UNITS", nullable = true) 055 private Long myHashIdentitySystemAndUnits; 056 057 /** 058 * Constructor 059 */ 060 public BaseResourceIndexedSearchParamQuantity() { 061 super(); 062 } 063 064 @Override 065 public void clearHashes() { 066 myHashIdentity = null; 067 myHashIdentityAndUnits = null; 068 myHashIdentitySystemAndUnits = null; 069 } 070 071 @Override 072 public void calculateHashes() { 073 if (myHashIdentity != null || myHashIdentityAndUnits != null || myHashIdentitySystemAndUnits != null) { 074 return; 075 } 076 077 String resourceType = getResourceType(); 078 String paramName = getParamName(); 079 String units = getUnits(); 080 String system = getSystem(); 081 setHashIdentity(calculateHashIdentity(getPartitionSettings(), getPartitionId(), resourceType, paramName)); 082 setHashIdentityAndUnits( 083 calculateHashUnits(getPartitionSettings(), getPartitionId(), resourceType, paramName, units)); 084 setHashIdentitySystemAndUnits(calculateHashSystemAndUnits( 085 getPartitionSettings(), getPartitionId(), resourceType, paramName, system, units)); 086 } 087 088 public Long getHashIdentityAndUnits() { 089 return myHashIdentityAndUnits; 090 } 091 092 public void setHashIdentityAndUnits(Long theHashIdentityAndUnits) { 093 myHashIdentityAndUnits = theHashIdentityAndUnits; 094 } 095 096 public Long getHashIdentitySystemAndUnits() { 097 return myHashIdentitySystemAndUnits; 098 } 099 100 public void setHashIdentitySystemAndUnits(Long theHashIdentitySystemAndUnits) { 101 myHashIdentitySystemAndUnits = theHashIdentitySystemAndUnits; 102 } 103 104 public String getSystem() { 105 return mySystem; 106 } 107 108 public void setSystem(String theSystem) { 109 mySystem = theSystem; 110 } 111 112 public String getUnits() { 113 return myUnits; 114 } 115 116 public void setUnits(String theUnits) { 117 myUnits = theUnits; 118 } 119 120 @Override 121 public int hashCode() { 122 HashCodeBuilder b = new HashCodeBuilder(); 123 b.append(getHashIdentity()); 124 b.append(getHashIdentityAndUnits()); 125 b.append(getHashIdentitySystemAndUnits()); 126 return b.toHashCode(); 127 } 128 129 public static long calculateHashSystemAndUnits( 130 PartitionSettings thePartitionSettings, 131 PartitionablePartitionId theRequestPartitionId, 132 String theResourceType, 133 String theParamName, 134 String theSystem, 135 String theUnits) { 136 RequestPartitionId requestPartitionId = PartitionablePartitionId.toRequestPartitionId(theRequestPartitionId); 137 return calculateHashSystemAndUnits( 138 thePartitionSettings, requestPartitionId, theResourceType, theParamName, theSystem, theUnits); 139 } 140 141 public static long calculateHashSystemAndUnits( 142 PartitionSettings thePartitionSettings, 143 RequestPartitionId theRequestPartitionId, 144 String theResourceType, 145 String theParamName, 146 String theSystem, 147 String theUnits) { 148 return hashSearchParam( 149 thePartitionSettings, theRequestPartitionId, theResourceType, theParamName, theSystem, theUnits); 150 } 151 152 public static long calculateHashUnits( 153 PartitionSettings thePartitionSettings, 154 PartitionablePartitionId theRequestPartitionId, 155 String theResourceType, 156 String theParamName, 157 String theUnits) { 158 RequestPartitionId requestPartitionId = PartitionablePartitionId.toRequestPartitionId(theRequestPartitionId); 159 return calculateHashUnits(thePartitionSettings, requestPartitionId, theResourceType, theParamName, theUnits); 160 } 161 162 public static long calculateHashUnits( 163 PartitionSettings thePartitionSettings, 164 RequestPartitionId theRequestPartitionId, 165 String theResourceType, 166 String theParamName, 167 String theUnits) { 168 return hashSearchParam(thePartitionSettings, theRequestPartitionId, theResourceType, theParamName, theUnits); 169 } 170}