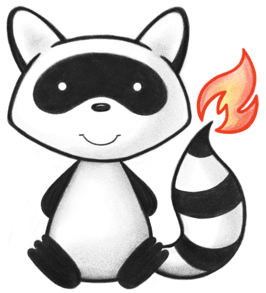
001/*- 002 * #%L 003 * HAPI FHIR JPA Model 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.model.entity; 021 022import jakarta.persistence.Column; 023import jakarta.persistence.Entity; 024import jakarta.persistence.Id; 025import jakarta.persistence.Lob; 026import jakarta.persistence.Table; 027import jakarta.persistence.Temporal; 028import jakarta.persistence.TemporalType; 029import org.hibernate.Length; 030 031import java.sql.Blob; 032import java.util.Date; 033 034import static java.util.Objects.nonNull; 035 036@Entity 037@Table(name = "HFJ_BINARY_STORAGE_BLOB") 038public class BinaryStorageEntity { 039 040 @Id 041 @Column(name = "BLOB_ID", length = 200, nullable = false) 042 // N.B GGG: Note that the `content id` is the same as the `externalized binary id`. 043 private String myContentId; 044 045 @Column(name = "RESOURCE_ID", length = 100, nullable = false) 046 private String myResourceId; 047 048 @Column(name = "BLOB_SIZE", nullable = false) 049 private long mySize; 050 051 @Column(name = "CONTENT_TYPE", nullable = false, length = 100) 052 private String myContentType; 053 054 /** 055 * @deprecated 056 */ 057 @Deprecated(since = "7.2.0") 058 @Lob // TODO: VC column added in 7.2.0 - Remove non-VC column later 059 @Column(name = "BLOB_DATA", nullable = true, insertable = true, updatable = false) 060 private Blob myBlob; 061 062 @Column(name = "STORAGE_CONTENT_BIN", nullable = true, length = Length.LONG32) 063 private byte[] myStorageContentBin; 064 065 @Temporal(TemporalType.TIMESTAMP) 066 @Column(name = "PUBLISHED_DATE", nullable = false) 067 private Date myPublished; 068 069 @Column(name = "BLOB_HASH", length = 128, nullable = true) 070 private String myHash; 071 072 public Date getPublished() { 073 return new Date(myPublished.getTime()); 074 } 075 076 public void setPublished(Date thePublishedDate) { 077 myPublished = thePublishedDate; 078 } 079 080 public String getHash() { 081 return myHash; 082 } 083 084 public void setContentId(String theContentId) { 085 myContentId = theContentId; 086 } 087 088 public void setResourceId(String theResourceId) { 089 myResourceId = theResourceId; 090 } 091 092 public long getSize() { 093 return mySize; 094 } 095 096 public String getContentType() { 097 return myContentType; 098 } 099 100 public void setContentType(String theContentType) { 101 myContentType = theContentType; 102 } 103 104 public Blob getBlob() { 105 return myBlob; 106 } 107 108 public void setBlob(Blob theBlob) { 109 myBlob = theBlob; 110 } 111 112 public String getContentId() { 113 return myContentId; 114 } 115 116 public void setSize(long theSize) { 117 mySize = theSize; 118 } 119 120 public void setHash(String theHash) { 121 myHash = theHash; 122 } 123 124 public byte[] getStorageContentBin() { 125 return myStorageContentBin; 126 } 127 128 public BinaryStorageEntity setStorageContentBin(byte[] theStorageContentBin) { 129 myStorageContentBin = theStorageContentBin; 130 return this; 131 } 132 133 public boolean hasStorageContent() { 134 return nonNull(myStorageContentBin); 135 } 136 137 public boolean hasBlob() { 138 return nonNull(myBlob); 139 } 140}