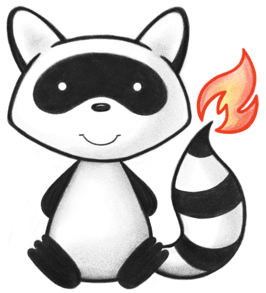
001package ca.uhn.fhir.jpa.model.entity; 002 003/*- 004 * #%L 005 * HAPI FHIR JPA Model 006 * %% 007 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 008 * %% 009 * Licensed under the Apache License, Version 2.0 (the "License"); 010 * you may not use this file except in compliance with the License. 011 * You may obtain a copy of the License at 012 * 013 * http://www.apache.org/licenses/LICENSE-2.0 014 * 015 * Unless required by applicable law or agreed to in writing, software 016 * distributed under the License is distributed on an "AS IS" BASIS, 017 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 018 * See the License for the specific language governing permissions and 019 * limitations under the License. 020 * #L% 021 */ 022 023import org.apache.commons.lang3.builder.ToStringBuilder; 024import org.hibernate.envers.RevisionType; 025 026import java.util.Date; 027import java.util.Objects; 028 029public class EnversRevision { 030 private final RevisionType myRevisionType; 031 private final long myRevisionNumber; 032 private final Date myRevisionTimestamp; 033 034 public EnversRevision(RevisionType theRevisionType, long theRevisionNumber, Date theRevisionTimestamp) { 035 myRevisionType = theRevisionType; 036 myRevisionNumber = theRevisionNumber; 037 myRevisionTimestamp = theRevisionTimestamp; 038 } 039 040 public RevisionType getRevisionType() { 041 return myRevisionType; 042 } 043 044 public long getRevisionNumber() { 045 return myRevisionNumber; 046 } 047 048 public Date getRevisionTimestamp() { 049 return myRevisionTimestamp; 050 } 051 052 @Override 053 public boolean equals(Object theO) { 054 055 if (this == theO) return true; 056 if (theO == null || getClass() != theO.getClass()) return false; 057 final EnversRevision that = (EnversRevision) theO; 058 return myRevisionNumber == that.myRevisionNumber 059 && myRevisionTimestamp == that.myRevisionTimestamp 060 && myRevisionType == that.myRevisionType; 061 } 062 063 @Override 064 public int hashCode() { 065 return Objects.hash(myRevisionType, myRevisionNumber, myRevisionTimestamp); 066 } 067 068 @Override 069 public String toString() { 070 return new ToStringBuilder(this) 071 .append("myRevisionType", myRevisionType) 072 .append("myRevisionNumber", myRevisionNumber) 073 .append("myRevisionTimestamp", myRevisionTimestamp) 074 .toString(); 075 } 076}