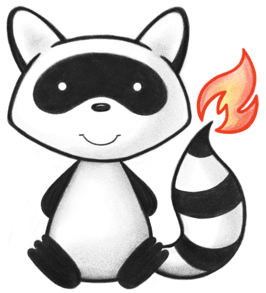
001/*- 002 * #%L 003 * HAPI FHIR JPA Model 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.model.entity; 021 022import org.apache.commons.lang3.builder.ToStringBuilder; 023import org.apache.commons.lang3.builder.ToStringStyle; 024 025import java.io.Serializable; 026import java.util.Objects; 027 028/** 029 * This class is used to store the PID for a given table, and if we're running in 030 * database partition mode, it also stores the partition ID. 031 */ 032public class IdAndPartitionId implements Serializable { 033 private Long myId; 034 private Integer myPartitionIdValue; 035 036 public IdAndPartitionId(Long theId, Integer thePartitionId) { 037 myId = theId; 038 myPartitionIdValue = thePartitionId; 039 } 040 041 public IdAndPartitionId() { 042 // nothing 043 } 044 045 public IdAndPartitionId(Long theId) { 046 myId = theId; 047 } 048 049 public Long getId() { 050 return myId; 051 } 052 053 public void setId(Long theId) { 054 myId = theId; 055 } 056 057 public void setPartitionIdValue(Integer thePartitionIdValue) { 058 myPartitionIdValue = thePartitionIdValue; 059 } 060 061 public Integer getPartitionIdValue() { 062 return myPartitionIdValue; 063 } 064 065 @Override 066 public boolean equals(Object theO) { 067 if (this == theO) return true; 068 if (!(theO instanceof IdAndPartitionId)) return false; 069 IdAndPartitionId that = (IdAndPartitionId) theO; 070 return Objects.equals(myId, that.myId) && Objects.equals(myPartitionIdValue, that.myPartitionIdValue); 071 } 072 073 @Override 074 public String toString() { 075 return new ToStringBuilder(this, ToStringStyle.SHORT_PREFIX_STYLE) 076 .append("id", myId) 077 .append("partitionId", myPartitionIdValue) 078 .toString(); 079 } 080 081 @Override 082 public int hashCode() { 083 return Objects.hash(myId, myPartitionIdValue); 084 } 085 086 public static IdAndPartitionId forId(Long theId, BasePartitionable thePartitionable) { 087 IdAndPartitionId retVal = new IdAndPartitionId(); 088 retVal.setId(theId); 089 retVal.setPartitionIdValue(thePartitionable.getPartitionId().getPartitionId()); 090 return retVal; 091 } 092}