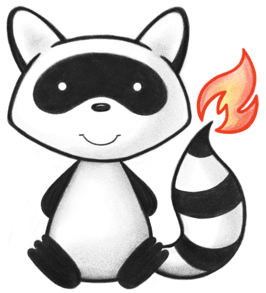
001/*- 002 * #%L 003 * HAPI FHIR JPA Model 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.model.entity; 021 022import ca.uhn.fhir.rest.api.Constants; 023import jakarta.persistence.Column; 024import jakarta.persistence.Entity; 025import jakarta.persistence.GeneratedValue; 026import jakarta.persistence.GenerationType; 027import jakarta.persistence.Id; 028import jakarta.persistence.SequenceGenerator; 029import jakarta.persistence.Table; 030import jakarta.persistence.UniqueConstraint; 031import org.hibernate.search.mapper.pojo.mapping.definition.annotation.FullTextField; 032 033/** 034 * Stores unique hash identities along with the corresponding {@code sp_name} and {@code res_type} values. 035 * This entity is populated during read, write, or update operations on the {@code HFJ_SPIDX_xxx} tables. 036 */ 037@Entity 038@Table( 039 name = "HFJ_SPIDX_IDENTITY", 040 uniqueConstraints = @UniqueConstraint(name = "IDX_HASH_IDENTITY", columnNames = "HASH_IDENTITY")) 041public class IndexedSearchParamIdentity { 042 043 public static final int SP_NAME_LENGTH = 256; 044 045 @Id 046 @SequenceGenerator(name = "SEQ_SPIDX_IDENTITY", sequenceName = "SEQ_SPIDX_IDENTITY", allocationSize = 1) 047 @GeneratedValue(strategy = GenerationType.AUTO, generator = "SEQ_SPIDX_IDENTITY") 048 @Column(name = "SP_IDENTITY_ID") 049 private Integer mySpIdentityId; 050 051 @Column(name = "HASH_IDENTITY", nullable = false) 052 private Long myHashIdentity; 053 054 @FullTextField 055 @Column(name = "RES_TYPE", nullable = false, length = Constants.MAX_RESOURCE_NAME_LENGTH) 056 private String myResourceType; 057 058 @FullTextField 059 @Column(name = "SP_NAME", nullable = false, length = SP_NAME_LENGTH) 060 private String myParamName; 061 062 public Integer getSpIdentityId() { 063 return mySpIdentityId; 064 } 065 066 public void setSpIdentityId(Integer theSpIdentityId) { 067 this.mySpIdentityId = theSpIdentityId; 068 } 069 070 public Long getHashIdentity() { 071 return myHashIdentity; 072 } 073 074 public void setHashIdentity(Long theHashIdentity) { 075 this.myHashIdentity = theHashIdentity; 076 } 077 078 public String getResourceType() { 079 return myResourceType; 080 } 081 082 public void setResourceType(String theResourceType) { 083 this.myResourceType = theResourceType; 084 } 085 086 public String getParamName() { 087 return myParamName; 088 } 089 090 public void setParamName(String theParamName) { 091 this.myParamName = theParamName; 092 } 093}