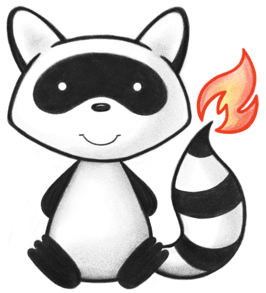
001/*- 002 * #%L 003 * HAPI FHIR JPA Model 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.model.entity; 021 022import jakarta.persistence.Column; 023import jakarta.persistence.Entity; 024import jakarta.persistence.GeneratedValue; 025import jakarta.persistence.GenerationType; 026import jakarta.persistence.Id; 027import jakarta.persistence.OneToMany; 028import jakarta.persistence.SequenceGenerator; 029import jakarta.persistence.Table; 030import jakarta.persistence.Temporal; 031import jakarta.persistence.TemporalType; 032import jakarta.persistence.UniqueConstraint; 033import jakarta.persistence.Version; 034import org.apache.commons.lang3.builder.EqualsBuilder; 035import org.apache.commons.lang3.builder.HashCodeBuilder; 036 037import java.util.Date; 038import java.util.List; 039 040@Entity() 041@Table( 042 name = "NPM_PACKAGE", 043 uniqueConstraints = {@UniqueConstraint(name = "IDX_PACK_ID", columnNames = "PACKAGE_ID")}) 044public class NpmPackageEntity { 045 046 protected static final int PACKAGE_ID_LENGTH = 200; 047 048 @SequenceGenerator(name = "SEQ_NPM_PACK", sequenceName = "SEQ_NPM_PACK") 049 @GeneratedValue(strategy = GenerationType.AUTO, generator = "SEQ_NPM_PACK") 050 @Id 051 @Column(name = "PID") 052 private Long myId; 053 054 @Column(name = "PACKAGE_ID", length = PACKAGE_ID_LENGTH, nullable = false) 055 private String myPackageId; 056 057 @Column(name = "CUR_VERSION_ID", length = NpmPackageVersionEntity.VERSION_ID_LENGTH, nullable = true) 058 private String myCurrentVersionId; 059 060 @Temporal(TemporalType.TIMESTAMP) 061 @Version 062 @Column(name = "UPDATED_TIME", nullable = false) 063 private Date myVersion; 064 065 @Column(name = "PACKAGE_DESC", length = NpmPackageVersionEntity.VERSION_ID_LENGTH, nullable = true) 066 private String myDescription; 067 068 @OneToMany(mappedBy = "myPackage") 069 private List<NpmPackageVersionEntity> myVersions; 070 071 public String getDescription() { 072 return myDescription; 073 } 074 075 public void setDescription(String theDescription) { 076 myDescription = theDescription; 077 } 078 079 public String getPackageId() { 080 return myPackageId; 081 } 082 083 public void setPackageId(String thePackageId) { 084 myPackageId = thePackageId; 085 } 086 087 @Override 088 public boolean equals(Object theO) { 089 if (this == theO) { 090 return true; 091 } 092 093 if (theO == null || getClass() != theO.getClass()) { 094 return false; 095 } 096 097 NpmPackageEntity that = (NpmPackageEntity) theO; 098 099 return new EqualsBuilder().append(myPackageId, that.myPackageId).isEquals(); 100 } 101 102 @Override 103 public int hashCode() { 104 return new HashCodeBuilder(17, 37).append(myPackageId).toHashCode(); 105 } 106 107 public String getCurrentVersionId() { 108 return myCurrentVersionId; 109 } 110 111 public void setCurrentVersionId(String theCurrentVersionId) { 112 myCurrentVersionId = theCurrentVersionId; 113 } 114}