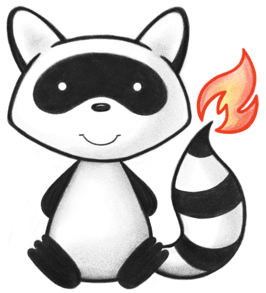
001/*- 002 * #%L 003 * HAPI FHIR JPA Model 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.model.entity; 021 022import ca.uhn.fhir.context.FhirVersionEnum; 023import jakarta.persistence.Column; 024import jakarta.persistence.Entity; 025import jakarta.persistence.EnumType; 026import jakarta.persistence.Enumerated; 027import jakarta.persistence.ForeignKey; 028import jakarta.persistence.GeneratedValue; 029import jakarta.persistence.GenerationType; 030import jakarta.persistence.Id; 031import jakarta.persistence.Index; 032import jakarta.persistence.JoinColumn; 033import jakarta.persistence.JoinColumns; 034import jakarta.persistence.ManyToOne; 035import jakarta.persistence.SequenceGenerator; 036import jakarta.persistence.Table; 037import jakarta.persistence.Temporal; 038import jakarta.persistence.TemporalType; 039import jakarta.persistence.Version; 040import org.apache.commons.lang3.builder.ToStringBuilder; 041import org.apache.commons.lang3.builder.ToStringStyle; 042import org.hibernate.annotations.JdbcTypeCode; 043import org.hibernate.type.SqlTypes; 044 045import java.util.Date; 046 047@Entity() 048@Table( 049 name = "NPM_PACKAGE_VER_RES", 050 indexes = { 051 @Index(name = "IDX_PACKVERRES_URL", columnList = "CANONICAL_URL"), 052 @Index(name = "FK_NPM_PACKVERRES_PACKVER", columnList = "PACKVER_PID"), 053 @Index(name = "FK_NPM_PKVR_RESID", columnList = "BINARY_RES_ID") 054 }) 055public class NpmPackageVersionResourceEntity { 056 057 @Id 058 @SequenceGenerator(name = "SEQ_NPM_PACKVERRES", sequenceName = "SEQ_NPM_PACKVERRES") 059 @GeneratedValue(strategy = GenerationType.AUTO, generator = "SEQ_NPM_PACKVERRES") 060 @Column(name = "PID") 061 private Long myId; 062 063 @ManyToOne 064 @JoinColumn( 065 name = "PACKVER_PID", 066 referencedColumnName = "PID", 067 foreignKey = @ForeignKey(name = "FK_NPM_PACKVERRES_PACKVER"), 068 nullable = false) 069 private NpmPackageVersionEntity myPackageVersion; 070 071 @ManyToOne 072 @JoinColumns( 073 value = { 074 @JoinColumn( 075 name = "BINARY_RES_ID", 076 referencedColumnName = "RES_ID", 077 nullable = false, 078 insertable = false, 079 updatable = false), 080 @JoinColumn( 081 name = "PARTITION_ID", 082 referencedColumnName = "PARTITION_ID", 083 nullable = false, 084 insertable = false, 085 updatable = false) 086 }, 087 foreignKey = @ForeignKey(name = "FK_NPM_PKVR_RESID")) 088 private ResourceTable myResourceBinary; 089 090 @Column(name = "BINARY_RES_ID", nullable = false) 091 private Long myResourcePid; 092 093 @Column(name = "PARTITION_ID", nullable = true) 094 private Integer myPartitionId; 095 096 @Column(name = "FILE_DIR", length = 200) 097 private String myDirectory; 098 099 @Column(name = "FILE_NAME", length = 200) 100 private String myFilename; 101 102 @Column(name = "RES_TYPE", length = ResourceTable.RESTYPE_LEN, nullable = false) 103 private String myResourceType; 104 105 @Column(name = "CANONICAL_URL", length = 200) 106 private String myCanonicalUrl; 107 108 @Column(name = "CANONICAL_VERSION", length = 200) 109 private String myCanonicalVersion; 110 111 @Enumerated(EnumType.STRING) 112 @JdbcTypeCode(SqlTypes.VARCHAR) 113 @Column(name = "FHIR_VERSION", length = NpmPackageVersionEntity.FHIR_VERSION_LENGTH, nullable = false) 114 private FhirVersionEnum myFhirVersion; 115 116 @Column(name = "FHIR_VERSION_ID", length = NpmPackageVersionEntity.FHIR_VERSION_ID_LENGTH, nullable = false) 117 private String myFhirVersionId; 118 119 @Column(name = "RES_SIZE_BYTES", nullable = false) 120 private long myResSizeBytes; 121 122 @Temporal(TemporalType.TIMESTAMP) 123 @Version 124 @Column(name = "UPDATED_TIME", nullable = false) 125 private Date myVersion; 126 127 public long getResSizeBytes() { 128 return myResSizeBytes; 129 } 130 131 public void setResSizeBytes(long theResSizeBytes) { 132 myResSizeBytes = theResSizeBytes; 133 } 134 135 public String getCanonicalVersion() { 136 return myCanonicalVersion; 137 } 138 139 public void setCanonicalVersion(String theCanonicalVersion) { 140 myCanonicalVersion = theCanonicalVersion; 141 } 142 143 public ResourceTable getResourceBinary() { 144 return myResourceBinary; 145 } 146 147 public void setResourceBinary(ResourceTable theResourceBinary) { 148 myResourceBinary = theResourceBinary; 149 myResourcePid = theResourceBinary.getId().getId(); 150 myPartitionId = theResourceBinary.getPersistentId().getPartitionId(); 151 } 152 153 public String getFhirVersionId() { 154 return myFhirVersionId; 155 } 156 157 public String getPackageId() { 158 return myPackageVersion.getPackageId(); 159 } 160 161 public String getPackageVersion() { 162 return myPackageVersion.getVersionId(); 163 } 164 165 public void setFhirVersionId(String theFhirVersionId) { 166 myFhirVersionId = theFhirVersionId; 167 } 168 169 public FhirVersionEnum getFhirVersion() { 170 return myFhirVersion; 171 } 172 173 public void setFhirVersion(FhirVersionEnum theFhirVersion) { 174 myFhirVersion = theFhirVersion; 175 } 176 177 public void setPackageVersion(NpmPackageVersionEntity thePackageVersion) { 178 myPackageVersion = thePackageVersion; 179 } 180 181 public String getDirectory() { 182 return myDirectory; 183 } 184 185 public void setDirectory(String theDirectory) { 186 myDirectory = theDirectory; 187 } 188 189 public String getFilename() { 190 return myFilename; 191 } 192 193 public void setFilename(String theFilename) { 194 myFilename = theFilename; 195 } 196 197 public String getResourceType() { 198 return myResourceType; 199 } 200 201 public void setResourceType(String theResourceType) { 202 myResourceType = theResourceType; 203 } 204 205 public String getCanonicalUrl() { 206 return myCanonicalUrl; 207 } 208 209 public void setCanonicalUrl(String theCanonicalUrl) { 210 myCanonicalUrl = theCanonicalUrl; 211 } 212 213 @Override 214 public String toString() { 215 216 return new ToStringBuilder(this, ToStringStyle.SHORT_PREFIX_STYLE) 217 .append("myId", myId) 218 .append("myCanonicalUrl", myCanonicalUrl) 219 .append("myCanonicalVersion", myCanonicalVersion) 220 .append("myResourceType", myResourceType) 221 .append("myDirectory", myDirectory) 222 .append("myFilename", myFilename) 223 .append("myPackageVersion", myPackageVersion) 224 .append("myResSizeBytes", myResSizeBytes) 225 .append("myVersion", myVersion) 226 .toString(); 227 } 228}