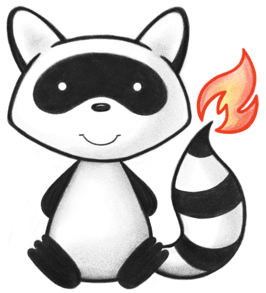
001/*- 002 * #%L 003 * HAPI FHIR JPA Model 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.model.entity; 021 022import ca.uhn.fhir.interceptor.model.RequestPartitionId; 023import ca.uhn.fhir.jpa.model.config.PartitionSettings; 024import jakarta.annotation.Nonnull; 025import jakarta.annotation.Nullable; 026import jakarta.persistence.Column; 027import jakarta.persistence.Embeddable; 028import org.apache.commons.lang3.builder.EqualsBuilder; 029import org.apache.commons.lang3.builder.HashCodeBuilder; 030 031import java.time.LocalDate; 032 033@Embeddable 034public class PartitionablePartitionId implements Cloneable { 035 036 public static final String PARTITION_ID = "PARTITION_ID"; 037 static final String PARTITION_DATE = "PARTITION_DATE"; 038 039 @Column(name = PARTITION_ID, nullable = true, insertable = true, updatable = false) 040 private Integer myPartitionId; 041 042 @Column(name = "PARTITION_DATE", nullable = true, insertable = true, updatable = false) 043 private LocalDate myPartitionDate; 044 045 /** 046 * Constructor 047 */ 048 public PartitionablePartitionId() { 049 super(); 050 } 051 052 /** 053 * Constructor 054 */ 055 public PartitionablePartitionId(@Nullable Integer thePartitionId, @Nullable LocalDate thePartitionDate) { 056 setPartitionId(thePartitionId); 057 setPartitionDate(thePartitionDate); 058 } 059 060 @Nullable 061 public Integer getPartitionId() { 062 return myPartitionId; 063 } 064 065 public PartitionablePartitionId setPartitionId(@Nullable Integer thePartitionId) { 066 myPartitionId = thePartitionId; 067 return this; 068 } 069 070 @Override 071 public boolean equals(Object theO) { 072 if (!(theO instanceof PartitionablePartitionId)) { 073 return false; 074 } 075 076 PartitionablePartitionId that = (PartitionablePartitionId) theO; 077 return new EqualsBuilder() 078 .append(myPartitionId, that.myPartitionId) 079 .append(myPartitionDate, that.myPartitionDate) 080 .isEquals(); 081 } 082 083 @Override 084 public int hashCode() { 085 return new HashCodeBuilder(17, 37) 086 .append(myPartitionId) 087 .append(myPartitionDate) 088 .toHashCode(); 089 } 090 091 @Nullable 092 public LocalDate getPartitionDate() { 093 return myPartitionDate; 094 } 095 096 public PartitionablePartitionId setPartitionDate(@Nullable LocalDate thePartitionDate) { 097 myPartitionDate = thePartitionDate; 098 return this; 099 } 100 101 @SuppressWarnings({"CloneDoesntDeclareCloneNotSupportedException", "MethodDoesntCallSuperMethod"}) 102 @Override 103 protected PartitionablePartitionId clone() { 104 return new PartitionablePartitionId().setPartitionId(getPartitionId()).setPartitionDate(getPartitionDate()); 105 } 106 107 public RequestPartitionId toPartitionId() { 108 return RequestPartitionId.fromPartitionId(getPartitionId(), getPartitionDate()); 109 } 110 111 @Override 112 public String toString() { 113 return "PartitionablePartitionId{" + "myPartitionId=" 114 + myPartitionId + ", myPartitionDate=" 115 + myPartitionDate + '}'; 116 } 117 118 @Nonnull 119 public static RequestPartitionId toRequestPartitionId(@Nullable PartitionablePartitionId theRequestPartitionId) { 120 if (theRequestPartitionId != null) { 121 return theRequestPartitionId.toPartitionId(); 122 } else { 123 return RequestPartitionId.defaultPartition(); 124 } 125 } 126 127 @Nonnull 128 public static PartitionablePartitionId toStoragePartition( 129 @Nonnull RequestPartitionId theRequestPartitionId, @Nonnull PartitionSettings thePartitionSettings) { 130 Integer partitionId = theRequestPartitionId.getFirstPartitionIdOrNull(); 131 if (partitionId == null) { 132 partitionId = thePartitionSettings.getDefaultPartitionId(); 133 } 134 return new PartitionablePartitionId(partitionId, theRequestPartitionId.getPartitionDate()); 135 } 136 137 public static PartitionablePartitionId with( 138 @Nullable Integer thePartitionId, @Nullable LocalDate thePartitionDate) { 139 return new PartitionablePartitionId(thePartitionId, thePartitionDate); 140 } 141}