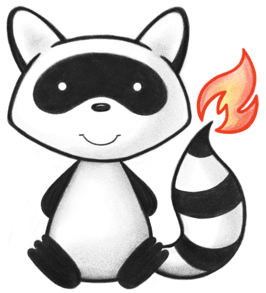
001/*- 002 * #%L 003 * HAPI FHIR JPA Model 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.model.entity; 021 022import ca.uhn.fhir.rest.api.Constants; 023import jakarta.persistence.Column; 024import jakarta.persistence.Entity; 025import jakarta.persistence.FetchType; 026import jakarta.persistence.ForeignKey; 027import jakarta.persistence.Id; 028import jakarta.persistence.Index; 029import jakarta.persistence.JoinColumn; 030import jakarta.persistence.ManyToOne; 031import jakarta.persistence.MapsId; 032import jakarta.persistence.OneToOne; 033import jakarta.persistence.Table; 034import org.apache.commons.lang3.builder.ToStringBuilder; 035import org.apache.commons.lang3.builder.ToStringStyle; 036 037import static ca.uhn.fhir.jpa.model.entity.ResourceHistoryTable.SOURCE_URI_LENGTH; 038 039/** 040 * This entity is deprecated - It stores the source URI and Request ID 041 * fields so that they can be indexed and searched discretely. In 042 * HAPI FHIR 6.8.0 we added equivalent columns to {@link ResourceHistoryTable} 043 * and started populating both those columns and the ones in this table. 044 * As of HAPI FHIR 8.0.0 we are no longer using this table unless 045 * the "AccessMetaSourceInformationFromProvenanceTable" on JpaStorageSettings 046 * is enabled (it's disabled by default). In the future we will remove 047 * this table entirely. 048 */ 049@Table( 050 name = "HFJ_RES_VER_PROV", 051 indexes = { 052 @Index(name = "IDX_RESVERPROV_SOURCEURI", columnList = "SOURCE_URI"), 053 @Index(name = "IDX_RESVERPROV_REQUESTID", columnList = "REQUEST_ID"), 054 @Index(name = "IDX_RESVERPROV_RES_PID", columnList = "RES_PID") 055 }) 056@Entity 057public class ResourceHistoryProvenanceEntity extends BasePartitionable { 058 059 @Id 060 @Column(name = "RES_VER_PID") 061 private Long myId; 062 063 @OneToOne(fetch = FetchType.LAZY) 064 @JoinColumn( 065 name = "RES_VER_PID", 066 referencedColumnName = "PID", 067 foreignKey = @ForeignKey(name = "FK_RESVERPROV_RESVER_PID"), 068 nullable = false, 069 insertable = false, 070 updatable = false) 071 @MapsId 072 private ResourceHistoryTable myResourceHistoryTable; 073 074 @ManyToOne(fetch = FetchType.LAZY) 075 @JoinColumn( 076 name = "RES_PID", 077 referencedColumnName = "RES_ID", 078 foreignKey = @ForeignKey(name = "FK_RESVERPROV_RES_PID"), 079 nullable = false) 080 private ResourceTable myResourceTable; 081 082 @Column(name = "SOURCE_URI", length = SOURCE_URI_LENGTH, nullable = true) 083 private String mySourceUri; 084 085 @Column(name = "REQUEST_ID", length = Constants.REQUEST_ID_LENGTH, nullable = true) 086 private String myRequestId; 087 088 /** 089 * Constructor 090 */ 091 public ResourceHistoryProvenanceEntity() { 092 super(); 093 } 094 095 @Override 096 public String toString() { 097 ToStringBuilder b = new ToStringBuilder(this, ToStringStyle.SHORT_PREFIX_STYLE); 098 b.append("resourceId", myResourceTable.getId()); 099 b.append("sourceUri", mySourceUri); 100 b.append("requestId", myRequestId); 101 return b.toString(); 102 } 103 104 public void setResourceTable(ResourceTable theResourceTable) { 105 myResourceTable = theResourceTable; 106 } 107 108 public void setResourceHistoryTable(ResourceHistoryTable theResourceHistoryTable) { 109 myResourceHistoryTable = theResourceHistoryTable; 110 } 111 112 public String getSourceUri() { 113 return mySourceUri; 114 } 115 116 public void setSourceUri(String theSourceUri) { 117 mySourceUri = theSourceUri; 118 } 119 120 public String getRequestId() { 121 return myRequestId; 122 } 123 124 public void setRequestId(String theRequestId) { 125 myRequestId = theRequestId; 126 } 127 128 public Long getId() { 129 return myId; 130 } 131}