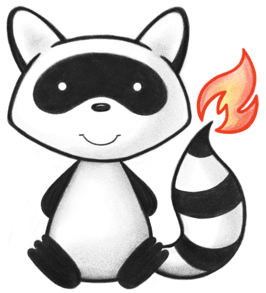
001/*- 002 * #%L 003 * HAPI FHIR JPA Model 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.model.entity; 021 022import jakarta.persistence.*; 023import org.apache.commons.lang3.Validate; 024import org.apache.commons.lang3.builder.CompareToBuilder; 025import org.apache.commons.lang3.builder.EqualsBuilder; 026import org.apache.commons.lang3.builder.HashCodeBuilder; 027import org.apache.commons.lang3.builder.ToStringBuilder; 028import org.apache.commons.lang3.builder.ToStringStyle; 029import org.hl7.fhir.instance.model.api.IIdType; 030 031@Entity() 032@Table( 033 name = "HFJ_IDX_CMP_STRING_UNIQ", 034 indexes = { 035 @Index( 036 name = ResourceIndexedComboStringUnique.IDX_IDXCMPSTRUNIQ_STRING, 037 columnList = "IDX_STRING", 038 unique = true), 039 @Index( 040 name = ResourceIndexedComboStringUnique.IDX_IDXCMPSTRUNIQ_RESOURCE, 041 columnList = "RES_ID", 042 unique = false) 043 }) 044public class ResourceIndexedComboStringUnique extends BasePartitionable 045 implements Comparable<ResourceIndexedComboStringUnique>, IResourceIndexComboSearchParameter { 046 047 public static final int MAX_STRING_LENGTH = 500; 048 public static final String IDX_IDXCMPSTRUNIQ_STRING = "IDX_IDXCMPSTRUNIQ_STRING"; 049 public static final String IDX_IDXCMPSTRUNIQ_RESOURCE = "IDX_IDXCMPSTRUNIQ_RESOURCE"; 050 051 @SequenceGenerator(name = "SEQ_IDXCMPSTRUNIQ_ID", sequenceName = "SEQ_IDXCMPSTRUNIQ_ID") 052 @GeneratedValue(strategy = GenerationType.AUTO, generator = "SEQ_IDXCMPSTRUNIQ_ID") 053 @Id 054 @Column(name = "PID") 055 private Long myId; 056 057 @ManyToOne 058 @JoinColumn( 059 name = "RES_ID", 060 referencedColumnName = "RES_ID", 061 foreignKey = @ForeignKey(name = "FK_IDXCMPSTRUNIQ_RES_ID")) 062 private ResourceTable myResource; 063 064 @Column(name = "RES_ID", insertable = false, updatable = false) 065 private Long myResourceId; 066 067 @Column(name = "IDX_STRING", nullable = false, length = MAX_STRING_LENGTH) 068 private String myIndexString; 069 070 /** 071 * This is here to support queries only, do not set this field directly 072 */ 073 @SuppressWarnings("unused") 074 @Column(name = PartitionablePartitionId.PARTITION_ID, insertable = false, updatable = false, nullable = true) 075 private Integer myPartitionIdValue; 076 077 @Transient 078 private IIdType mySearchParameterId; 079 080 /** 081 * Constructor 082 */ 083 public ResourceIndexedComboStringUnique() { 084 super(); 085 } 086 087 /** 088 * Constructor 089 */ 090 public ResourceIndexedComboStringUnique( 091 ResourceTable theResource, String theIndexString, IIdType theSearchParameterId) { 092 setResource(theResource); 093 setIndexString(theIndexString); 094 setPartitionId(theResource.getPartitionId()); 095 setSearchParameterId(theSearchParameterId); 096 } 097 098 @Override 099 public int compareTo(ResourceIndexedComboStringUnique theO) { 100 CompareToBuilder b = new CompareToBuilder(); 101 b.append(myIndexString, theO.getIndexString()); 102 return b.toComparison(); 103 } 104 105 @Override 106 public boolean equals(Object theO) { 107 if (this == theO) return true; 108 109 if (!(theO instanceof ResourceIndexedComboStringUnique)) { 110 return false; 111 } 112 113 ResourceIndexedComboStringUnique that = (ResourceIndexedComboStringUnique) theO; 114 115 return new EqualsBuilder().append(myIndexString, that.myIndexString).isEquals(); 116 } 117 118 @Override 119 public String getIndexString() { 120 return myIndexString; 121 } 122 123 public void setIndexString(String theIndexString) { 124 myIndexString = theIndexString; 125 } 126 127 public ResourceTable getResource() { 128 return myResource; 129 } 130 131 public void setResource(ResourceTable theResource) { 132 Validate.notNull(theResource); 133 myResource = theResource; 134 } 135 136 @Override 137 public int hashCode() { 138 return new HashCodeBuilder(17, 37).append(myIndexString).toHashCode(); 139 } 140 141 @Override 142 public String toString() { 143 return new ToStringBuilder(this, ToStringStyle.SHORT_PREFIX_STYLE) 144 .append("id", myId) 145 .append("resourceId", myResourceId) 146 .append("indexString", myIndexString) 147 .append("partition", getPartitionId()) 148 .toString(); 149 } 150 151 /** 152 * Note: This field is not persisted, so it will only be populated for new indexes 153 */ 154 @Override 155 public void setSearchParameterId(IIdType theSearchParameterId) { 156 mySearchParameterId = theSearchParameterId; 157 } 158 159 /** 160 * Note: This field is not persisted, so it will only be populated for new indexes 161 */ 162 @Override 163 public IIdType getSearchParameterId() { 164 return mySearchParameterId; 165 } 166}