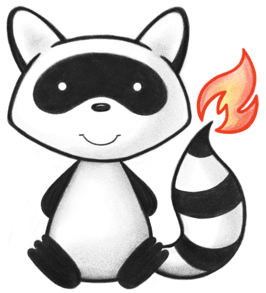
001/*- 002 * #%L 003 * HAPI FHIR JPA Model 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.model.entity; 021 022import jakarta.persistence.Column; 023import jakarta.persistence.Embeddable; 024 025import java.io.Serializable; 026import java.util.Objects; 027import java.util.Optional; 028import java.util.StringJoiner; 029 030/** 031 * Multi-column primary Key for {@link ResourceSearchUrlEntity} 032 */ 033@Embeddable 034public class ResourceSearchUrlEntityPK implements Serializable { 035 public static final String RES_SEARCH_URL_COLUMN_NAME = "RES_SEARCH_URL"; 036 public static final String PARTITION_ID_COLUMN_NAME = "PARTITION_ID"; 037 038 public static final int RES_SEARCH_URL_LENGTH = 768; 039 040 private static final long serialVersionUID = 1L; 041 042 private static final int PARTITION_ID_NULL_EQUIVALENT = -1; 043 044 @Column(name = RES_SEARCH_URL_COLUMN_NAME, length = RES_SEARCH_URL_LENGTH, nullable = false) 045 // Weird field name isto ensure that this the first key in the index 046 private String my_A_SearchUrl; 047 048 @Column(name = PARTITION_ID_COLUMN_NAME, nullable = false, insertable = false, updatable = false) 049 // Weird field name isto ensure that this the second key in the index 050 private Integer my_B_PartitionId; 051 052 public ResourceSearchUrlEntityPK() {} 053 054 public static ResourceSearchUrlEntityPK from( 055 String theSearchUrl, ResourceTable theResourceTable, boolean theSearchUrlDuplicateAcrossPartitionsEnabled) { 056 return new ResourceSearchUrlEntityPK( 057 theSearchUrl, 058 computePartitionIdOrNullEquivalent(theResourceTable, theSearchUrlDuplicateAcrossPartitionsEnabled)); 059 } 060 061 public ResourceSearchUrlEntityPK(String theSearchUrl, int thePartitionId) { 062 my_A_SearchUrl = theSearchUrl; 063 my_B_PartitionId = thePartitionId; 064 } 065 066 public String getSearchUrl() { 067 return my_A_SearchUrl; 068 } 069 070 public void setSearchUrl(String theMy_A_SearchUrl) { 071 my_A_SearchUrl = theMy_A_SearchUrl; 072 } 073 074 public Integer getPartitionId() { 075 return my_B_PartitionId; 076 } 077 078 public void setPartitionId(Integer theMy_B_PartitionId) { 079 my_B_PartitionId = theMy_B_PartitionId; 080 } 081 082 @Override 083 public boolean equals(Object theO) { 084 if (this == theO) { 085 return true; 086 } 087 if (theO == null || getClass() != theO.getClass()) { 088 return false; 089 } 090 ResourceSearchUrlEntityPK that = (ResourceSearchUrlEntityPK) theO; 091 return Objects.equals(my_A_SearchUrl, that.my_A_SearchUrl) 092 && Objects.equals(my_B_PartitionId, that.my_B_PartitionId); 093 } 094 095 @Override 096 public int hashCode() { 097 return Objects.hash(my_A_SearchUrl, my_B_PartitionId); 098 } 099 100 @Override 101 public String toString() { 102 return new StringJoiner(", ", ResourceSearchUrlEntityPK.class.getSimpleName() + "[", "]") 103 .add("my_A_SearchUrl='" + my_A_SearchUrl + "'") 104 .add("my_B_PartitionId=" + my_B_PartitionId) 105 .toString(); 106 } 107 108 private static int computePartitionIdOrNullEquivalent( 109 ResourceTable theResourceTable, boolean theSearchUrlDuplicateAcrossPartitionsEnabled) { 110 if (!theSearchUrlDuplicateAcrossPartitionsEnabled) { 111 return PARTITION_ID_NULL_EQUIVALENT; 112 } 113 114 return Optional.ofNullable(theResourceTable.getPartitionId()) 115 .map(PartitionablePartitionId::getPartitionId) 116 .orElse(PARTITION_ID_NULL_EQUIVALENT); 117 } 118}