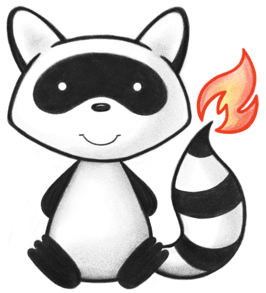
001/* 002 * #%L 003 * HAPI FHIR JPA Model 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.model.entity; 021 022import jakarta.persistence.Column; 023import jakarta.persistence.Entity; 024import jakarta.persistence.FetchType; 025import jakarta.persistence.ForeignKey; 026import jakarta.persistence.GeneratedValue; 027import jakarta.persistence.GenerationType; 028import jakarta.persistence.Id; 029import jakarta.persistence.Index; 030import jakarta.persistence.JoinColumn; 031import jakarta.persistence.ManyToOne; 032import jakarta.persistence.SequenceGenerator; 033import jakarta.persistence.Table; 034import jakarta.persistence.UniqueConstraint; 035import org.apache.commons.lang3.builder.EqualsBuilder; 036import org.apache.commons.lang3.builder.HashCodeBuilder; 037import org.apache.commons.lang3.builder.ToStringBuilder; 038import org.apache.commons.lang3.builder.ToStringStyle; 039 040@Entity 041@Table( 042 name = "HFJ_RES_TAG", 043 indexes = { 044 @Index(name = "IDX_RES_TAG_RES_TAG", columnList = "RES_ID, TAG_ID, PARTITION_ID"), 045 @Index(name = "IDX_RES_TAG_TAG_RES", columnList = "TAG_ID, RES_ID, PARTITION_ID") 046 }, 047 uniqueConstraints = { 048 @UniqueConstraint( 049 name = "IDX_RESTAG_TAGID", 050 columnNames = {"RES_ID", "TAG_ID"}) 051 }) 052public class ResourceTag extends BaseTag { 053 054 private static final long serialVersionUID = 1L; 055 056 @SequenceGenerator(name = "SEQ_RESTAG_ID", sequenceName = "SEQ_RESTAG_ID") 057 @GeneratedValue(strategy = GenerationType.AUTO, generator = "SEQ_RESTAG_ID") 058 @Id 059 @Column(name = "PID") 060 private Long myId; 061 062 @ManyToOne( 063 cascade = {}, 064 fetch = FetchType.LAZY) 065 @JoinColumn(name = "RES_ID", referencedColumnName = "RES_ID", foreignKey = @ForeignKey(name = "FK_RESTAG_RESOURCE")) 066 private ResourceTable myResource; 067 068 @Column(name = "RES_TYPE", length = ResourceTable.RESTYPE_LEN, nullable = false) 069 private String myResourceType; 070 071 @Column(name = "RES_ID", insertable = false, updatable = false) 072 private Long myResourceId; 073 074 /** 075 * Constructor 076 */ 077 public ResourceTag() { 078 super(); 079 } 080 081 /** 082 * Constructor 083 */ 084 public ResourceTag( 085 ResourceTable theResourceTable, TagDefinition theTag, PartitionablePartitionId theRequestPartitionId) { 086 setTag(theTag); 087 setResource(theResourceTable); 088 setResourceId(theResourceTable.getId()); 089 setResourceType(theResourceTable.getResourceType()); 090 setPartitionId(theRequestPartitionId); 091 } 092 093 public Long getResourceId() { 094 return myResourceId; 095 } 096 097 public void setResourceId(Long theResourceId) { 098 myResourceId = theResourceId; 099 } 100 101 public ResourceTable getResource() { 102 return myResource; 103 } 104 105 public void setResource(ResourceTable theResource) { 106 myResource = theResource; 107 } 108 109 public String getResourceType() { 110 return myResourceType; 111 } 112 113 public void setResourceType(String theResourceType) { 114 myResourceType = theResourceType; 115 } 116 117 @Override 118 public boolean equals(Object obj) { 119 if (this == obj) { 120 return true; 121 } 122 if (obj == null) { 123 return false; 124 } 125 if (!(obj instanceof ResourceTag)) { 126 return false; 127 } 128 ResourceTag other = (ResourceTag) obj; 129 EqualsBuilder b = new EqualsBuilder(); 130 b.append(getResourceId(), other.getResourceId()); 131 b.append(getTag(), other.getTag()); 132 return b.isEquals(); 133 } 134 135 @Override 136 public int hashCode() { 137 HashCodeBuilder b = new HashCodeBuilder(); 138 b.append(getResourceId()); 139 b.append(getTag()); 140 return b.toHashCode(); 141 } 142 143 @Override 144 public String toString() { 145 ToStringBuilder b = new ToStringBuilder(this, ToStringStyle.SHORT_PREFIX_STYLE); 146 if (getPartitionId() != null) { 147 b.append("partition", getPartitionId().getPartitionId()); 148 } 149 b.append("resId", getResourceId()); 150 b.append("tag", getTag().getId()); 151 return b.build(); 152 } 153}