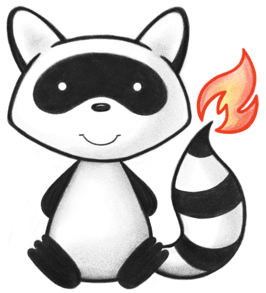
001/* 002 * #%L 003 * HAPI FHIR JPA Model 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.model.entity; 021 022import jakarta.persistence.Column; 023import jakarta.persistence.Entity; 024import jakarta.persistence.EnumType; 025import jakarta.persistence.Enumerated; 026import jakarta.persistence.FetchType; 027import jakarta.persistence.GeneratedValue; 028import jakarta.persistence.GenerationType; 029import jakarta.persistence.Id; 030import jakarta.persistence.Index; 031import jakarta.persistence.OneToMany; 032import jakarta.persistence.SequenceGenerator; 033import jakarta.persistence.Table; 034import jakarta.persistence.Transient; 035import org.apache.commons.lang3.StringUtils; 036import org.apache.commons.lang3.builder.EqualsBuilder; 037import org.apache.commons.lang3.builder.HashCodeBuilder; 038import org.apache.commons.lang3.builder.ToStringBuilder; 039import org.apache.commons.lang3.builder.ToStringStyle; 040import org.hibernate.annotations.JdbcTypeCode; 041import org.hibernate.type.SqlTypes; 042 043import java.io.Serializable; 044import java.util.Collection; 045 046@Entity 047@Table( 048 name = "HFJ_TAG_DEF", 049 indexes = { 050 @Index( 051 name = "IDX_TAG_DEF_TP_CD_SYS", 052 columnList = "TAG_TYPE, TAG_CODE, TAG_SYSTEM, TAG_ID, TAG_VERSION, TAG_USER_SELECTED"), 053 }) 054public class TagDefinition implements Serializable { 055 056 private static final long serialVersionUID = 1L; 057 058 @Column(name = "TAG_CODE", length = 200) 059 private String myCode; 060 061 @Column(name = "TAG_DISPLAY", length = 200) 062 private String myDisplay; 063 064 @Id 065 @GeneratedValue(strategy = GenerationType.AUTO, generator = "SEQ_TAGDEF_ID") 066 @SequenceGenerator(name = "SEQ_TAGDEF_ID", sequenceName = "SEQ_TAGDEF_ID") 067 @Column(name = "TAG_ID") 068 private Long myId; 069 070 // one tag definition -> many resource tags 071 @OneToMany( 072 cascade = {}, 073 fetch = FetchType.LAZY, 074 mappedBy = "myTag") 075 private Collection<ResourceTag> myResources; 076 077 // one tag definition -> many history 078 @OneToMany( 079 cascade = {}, 080 fetch = FetchType.LAZY, 081 mappedBy = "myTag") 082 private Collection<ResourceHistoryTag> myResourceVersions; 083 084 @Column(name = "TAG_SYSTEM", length = 200) 085 private String mySystem; 086 087 @Column(name = "TAG_TYPE", nullable = false) 088 @Enumerated(EnumType.ORDINAL) 089 @JdbcTypeCode(SqlTypes.INTEGER) 090 private TagTypeEnum myTagType; 091 092 @Column(name = "TAG_VERSION", length = 30) 093 private String myVersion; 094 095 @Column(name = "TAG_USER_SELECTED") 096 private Boolean myUserSelected; 097 098 @Transient 099 private transient Integer myHashCode; 100 101 /** 102 * Constructor 103 */ 104 public TagDefinition() { 105 super(); 106 } 107 108 public TagDefinition(TagTypeEnum theTagType, String theSystem, String theCode, String theDisplay) { 109 setTagType(theTagType); 110 setCode(theCode); 111 setSystem(theSystem); 112 setDisplay(theDisplay); 113 } 114 115 public String getCode() { 116 return myCode; 117 } 118 119 public void setCode(String theCode) { 120 myCode = theCode; 121 myHashCode = null; 122 } 123 124 public String getDisplay() { 125 return myDisplay; 126 } 127 128 public void setDisplay(String theDisplay) { 129 myDisplay = theDisplay; 130 } 131 132 public Long getId() { 133 return myId; 134 } 135 136 public void setId(Long theId) { 137 myId = theId; 138 } 139 140 public String getSystem() { 141 return mySystem; 142 } 143 144 public void setSystem(String theSystem) { 145 mySystem = theSystem; 146 myHashCode = null; 147 } 148 149 public TagTypeEnum getTagType() { 150 return myTagType; 151 } 152 153 public void setTagType(TagTypeEnum theTagType) { 154 myTagType = theTagType; 155 myHashCode = null; 156 } 157 158 public String getVersion() { 159 return myVersion; 160 } 161 162 public void setVersion(String theVersion) { 163 setVersionAfterTrim(theVersion); 164 } 165 166 private void setVersionAfterTrim(String theVersion) { 167 if (theVersion != null) { 168 myVersion = StringUtils.truncate(theVersion, 30); 169 } 170 } 171 172 /** 173 * Warning - this is nullable, while IBaseCoding getUserSelected isn't. 174 * todo maybe rename? 175 */ 176 public Boolean getUserSelected() { 177 return myUserSelected; 178 } 179 180 public void setUserSelected(Boolean theUserSelected) { 181 myUserSelected = theUserSelected; 182 } 183 184 @Override 185 public boolean equals(Object obj) { 186 if (this == obj) { 187 return true; 188 } 189 if (!(obj instanceof TagDefinition)) { 190 return false; 191 } 192 TagDefinition other = (TagDefinition) obj; 193 194 EqualsBuilder b = new EqualsBuilder(); 195 b.append(myTagType, other.myTagType); 196 b.append(mySystem, other.mySystem); 197 b.append(myCode, other.myCode); 198 b.append(myVersion, other.myVersion); 199 b.append(myUserSelected, other.myUserSelected); 200 return b.isEquals(); 201 } 202 203 @Override 204 public int hashCode() { 205 if (myHashCode == null) { 206 HashCodeBuilder b = new HashCodeBuilder(); 207 b.append(myTagType); 208 b.append(mySystem); 209 b.append(myCode); 210 b.append(myVersion); 211 b.append(myUserSelected); 212 myHashCode = b.toHashCode(); 213 } 214 return myHashCode; 215 } 216 217 @Override 218 public String toString() { 219 ToStringBuilder retVal = new ToStringBuilder(this, ToStringStyle.SHORT_PREFIX_STYLE); 220 retVal.append("id", myId); 221 retVal.append("system", mySystem); 222 retVal.append("code", myCode); 223 retVal.append("display", myDisplay); 224 retVal.append("version", myVersion); 225 retVal.append("userSelected", myUserSelected); 226 return retVal.build(); 227 } 228}