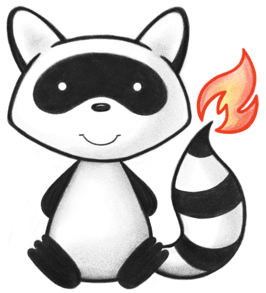
001/*- 002 * #%L 003 * HAPI FHIR JPA Model 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.model.search; 021 022import ca.uhn.fhir.jpa.model.entity.EntityIndexStatusEnum; 023import ca.uhn.fhir.jpa.model.entity.ResourceTable; 024import org.hibernate.search.mapper.pojo.bridge.RoutingBridge; 025import org.hibernate.search.mapper.pojo.bridge.binding.RoutingBindingContext; 026import org.hibernate.search.mapper.pojo.bridge.mapping.programmatic.RoutingBinder; 027import org.hibernate.search.mapper.pojo.bridge.runtime.RoutingBridgeRouteContext; 028import org.hibernate.search.mapper.pojo.route.DocumentRoutes; 029 030public class ResourceTableRoutingBinder implements RoutingBinder { 031 @Override 032 public void bind(RoutingBindingContext theRoutingBindingContext) { 033 theRoutingBindingContext.dependencies().use("myDeleted").use("myIndexStatus"); 034 theRoutingBindingContext.bridge(ResourceTable.class, new ResourceTableBridge()); 035 } 036 037 private static class ResourceTableBridge implements RoutingBridge<ResourceTable> { 038 039 @Override 040 public void route( 041 DocumentRoutes theDocumentRoutes, 042 Object theO, 043 ResourceTable theResourceTable, 044 RoutingBridgeRouteContext theRoutingBridgeRouteContext) { 045 if (theResourceTable.getDeleted() == null 046 && theResourceTable.getIndexStatus() == EntityIndexStatusEnum.INDEXED_ALL) { 047 theDocumentRoutes.addRoute(); 048 } else { 049 theDocumentRoutes.notIndexed(); 050 } 051 } 052 053 @Override 054 public void previousRoutes( 055 DocumentRoutes theDocumentRoutes, 056 Object theO, 057 ResourceTable theResourceTable, 058 RoutingBridgeRouteContext theRoutingBridgeRouteContext) { 059 theDocumentRoutes.addRoute(); 060 } 061 } 062}