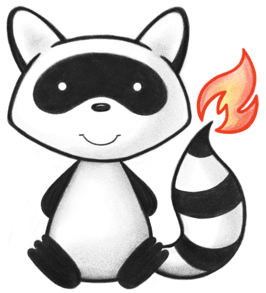
001/*- 002 * #%L 003 * HAPI FHIR JPA Model 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.model.util; 021 022import ca.uhn.fhir.rest.api.Constants; 023import ca.uhn.fhir.rest.server.provider.ProviderConstants; 024import ca.uhn.fhir.util.HapiExtensions; 025 026import java.util.Set; 027 028public class JpaConstants { 029 030 /** 031 * Userdata key for tracking the fact that a resource ID was assigned by the server 032 */ 033 public static final String RESOURCE_ID_SERVER_ASSIGNED = 034 JpaConstants.class.getName() + "_RESOURCE_ID_SERVER_ASSIGNED"; 035 /** 036 * Operation name for the $apply-codesystem-delta-add operation 037 */ 038 public static final String OPERATION_APPLY_CODESYSTEM_DELTA_ADD = "$apply-codesystem-delta-add"; 039 /** 040 * Operation name for the $apply-codesystem-delta-remove operation 041 */ 042 public static final String OPERATION_APPLY_CODESYSTEM_DELTA_REMOVE = "$apply-codesystem-delta-remove"; 043 /** 044 * Operation name for the $expunge operation 045 * 046 * @deprecated Replace with {@link ProviderConstants#OPERATION_EXPUNGE} 047 */ 048 @Deprecated 049 public static final String OPERATION_EXPUNGE = ProviderConstants.OPERATION_EXPUNGE; 050 /** 051 * @deprecated Replace with {@link ProviderConstants#OPERATION_EXPUNGE} 052 */ 053 @Deprecated 054 public static final String OPERATION_NAME_EXPUNGE = ProviderConstants.OPERATION_EXPUNGE; 055 /** 056 * @deprecated Replace with {@link ProviderConstants#OPERATION_EXPUNGE_OUT_PARAM_EXPUNGE_COUNT} 057 */ 058 @Deprecated 059 public static final String OPERATION_EXPUNGE_PARAM_LIMIT = ProviderConstants.OPERATION_EXPUNGE_PARAM_LIMIT; 060 /** 061 * @deprecated Replace with {@link ProviderConstants#OPERATION_EXPUNGE_OUT_PARAM_EXPUNGE_COUNT} 062 */ 063 @Deprecated 064 public static final String OPERATION_EXPUNGE_PARAM_EXPUNGE_DELETED_RESOURCES = 065 ProviderConstants.OPERATION_EXPUNGE_PARAM_EXPUNGE_DELETED_RESOURCES; 066 /** 067 * @deprecated Replace with {@link ProviderConstants#OPERATION_EXPUNGE_OUT_PARAM_EXPUNGE_COUNT} 068 */ 069 @Deprecated 070 public static final String OPERATION_EXPUNGE_PARAM_EXPUNGE_PREVIOUS_VERSIONS = 071 ProviderConstants.OPERATION_EXPUNGE_PARAM_EXPUNGE_PREVIOUS_VERSIONS; 072 /** 073 * @deprecated Replace with {@link ProviderConstants#OPERATION_EXPUNGE_OUT_PARAM_EXPUNGE_COUNT} 074 */ 075 @Deprecated 076 public static final String OPERATION_EXPUNGE_PARAM_EXPUNGE_EVERYTHING = 077 ProviderConstants.OPERATION_EXPUNGE_PARAM_EXPUNGE_EVERYTHING; 078 /** 079 * @deprecated Replace with {@link ProviderConstants#OPERATION_EXPUNGE_OUT_PARAM_EXPUNGE_COUNT} 080 */ 081 @Deprecated 082 public static final String OPERATION_EXPUNGE_OUT_PARAM_EXPUNGE_COUNT = 083 ProviderConstants.OPERATION_EXPUNGE_OUT_PARAM_EXPUNGE_COUNT; 084 /** 085 * Header name for the "X-Meta-Snapshot-Mode" header, which 086 * specifies that properties in meta (tags, profiles, security labels) 087 * should be treated as a snapshot, meaning that these things will 088 * be removed if they are not explicitly included in updates 089 */ 090 public static final String HEADER_META_SNAPSHOT_MODE = "X-Meta-Snapshot-Mode"; 091 /** 092 * Operation name for the $lookup operation 093 */ 094 public static final String OPERATION_LOOKUP = "$lookup"; 095 /** 096 * Operation name for the $expand operation 097 */ 098 public static final String OPERATION_EXPAND = "$expand"; 099 /** 100 * Operation name for the $validate-code operation 101 */ 102 public static final String OPERATION_VALIDATE_CODE = "$validate-code"; 103 /** 104 * Operation name for the $get-resource-counts operation 105 */ 106 public static final String OPERATION_GET_RESOURCE_COUNTS = "$get-resource-counts"; 107 /** 108 * Operation name for the $validate operation 109 */ 110 // NB don't delete this, it's used in Smile as well, even though hapi-fhir-server uses the version from 111 // Constants.java 112 public static final String OPERATION_VALIDATE = Constants.EXTOP_VALIDATE; 113 /** 114 * Operation name for the $everything operation 115 */ 116 public static final String OPERATION_EVERYTHING = "$everything"; 117 /** 118 * Operation name for the $process-message operation 119 */ 120 public static final String OPERATION_PROCESS_MESSAGE = "$process-message"; 121 /** 122 * Operation name for the $meta-delete operation 123 */ 124 public static final String OPERATION_META_DELETE = "$meta-delete"; 125 /** 126 * Operation name for the $meta-add operation 127 */ 128 public static final String OPERATION_META_ADD = "$meta-add"; 129 /** 130 * Operation name for the $translate operation 131 */ 132 public static final String OPERATION_TRANSLATE = "$translate"; 133 /** 134 * Operation name for the $document operation 135 */ 136 public static final String OPERATION_DOCUMENT = "$document"; 137 /** 138 * Trigger a subscription manually for a given resource 139 */ 140 public static final String OPERATION_TRIGGER_SUBSCRIPTION = "$trigger-subscription"; 141 /** 142 * Operation name for the "$subsumes" operation 143 */ 144 public static final String OPERATION_SUBSUMES = "$subsumes"; 145 /** 146 * Operation name for the "$snapshot" operation 147 */ 148 public static final String OPERATION_SNAPSHOT = "$snapshot"; 149 /** 150 * Operation name for the "$binary-access" operation 151 */ 152 public static final String OPERATION_BINARY_ACCESS_READ = "$binary-access-read"; 153 /** 154 * Operation name for the "$binary-access" operation 155 */ 156 public static final String OPERATION_BINARY_ACCESS_WRITE = "$binary-access-write"; 157 /** 158 * Operation name for the "$upload-external-code-system" operation 159 */ 160 public static final String OPERATION_UPLOAD_EXTERNAL_CODE_SYSTEM = "$upload-external-code-system"; 161 /** 162 * Operation name for the "$import" operation 163 */ 164 public static final String OPERATION_IMPORT = "$import"; 165 /** 166 * Operation name for the "$import-poll-status" operation 167 */ 168 public static final String OPERATION_IMPORT_POLL_STATUS = "$import-poll-status"; 169 /** 170 * Operation name for the "$lastn" operation 171 */ 172 public static final String OPERATION_LASTN = "$lastn"; 173 174 /** 175 * Operation name for the $member-match operation 176 */ 177 public static final String OPERATION_MEMBER_MATCH = "$member-match"; 178 179 /** 180 * Parameter for the $export operation 181 */ 182 public static final String PARAM_EXPORT_POLL_STATUS_JOB_ID = "_jobId"; 183 /** 184 * Parameter for the $export operation 185 */ 186 public static final String PARAM_EXPORT_OUTPUT_FORMAT = "_outputFormat"; 187 /** 188 * Parameter for the $export operation 189 */ 190 public static final String PARAM_EXPORT_TYPE = "_type"; 191 /** 192 * Parameter for the $export operation 193 */ 194 public static final String PARAM_EXPORT_SINCE = "_since"; 195 /** 196 * Parameter for the $export operation 197 */ 198 public static final String PARAM_EXPORT_TYPE_FILTER = "_typeFilter"; 199 200 /** 201 * Parameter for the $export operation to identify binaries with a given identifier. 202 */ 203 public static final String PARAM_EXPORT_IDENTIFIER = "_exportId"; 204 /** 205 * Parameter for the $export operation 206 */ 207 public static final String PARAM_EXPORT_TYPE_POST_FETCH_FILTER_URL = "_typePostFetchFilterUrl"; 208 /** 209 * Parameter for the $export operation 210 */ 211 public static final String PARAM_EXPORT_PATIENT = "patient"; 212 213 /** 214 * Parameter for the $import operation 215 */ 216 public static final String PARAM_IMPORT_POLL_STATUS_JOB_ID = "_jobId"; 217 /** 218 * Parameter for the $import operation 219 */ 220 public static final String PARAM_IMPORT_JOB_DESCRIPTION = "_jobDescription"; 221 /** 222 * Parameter for the $import operation 223 */ 224 public static final String PARAM_IMPORT_PROCESSING_MODE = "_processingMode"; 225 /** 226 * Parameter for the $import operation 227 */ 228 public static final String PARAM_IMPORT_FILE_COUNT = "_fileCount"; 229 /** 230 * Parameter for the $import operation 231 */ 232 public static final String PARAM_IMPORT_BATCH_SIZE = "_batchSize"; 233 234 /** 235 * The [id] of the group when $export is called on /Group/[id]/$export 236 */ 237 public static final String PARAM_EXPORT_GROUP_ID = "_groupId"; 238 239 /** 240 * Whether mdm should be performed on group export items to expand the group items to linked items before performing the export 241 */ 242 public static final String PARAM_EXPORT_MDM = "_mdm"; 243 244 /** 245 * Parameter for delete to indicate the deleted resources should also be expunged 246 */ 247 public static final String PARAM_DELETE_EXPUNGE = "_expunge"; 248 249 /** 250 * URL for extension on a SearchParameter indicating that text values should not be indexed 251 */ 252 public static final String EXTENSION_EXT_SYSTEMDEFINED = 253 JpaConstants.class.getName() + "_EXTENSION_EXT_SYSTEMDEFINED"; 254 255 /** 256 * Deprecated. Please use {@link HapiExtensions#EXT_SEARCHPARAM_PHONETIC_ENCODER} instead. 257 */ 258 @Deprecated 259 public static final String EXT_SEARCHPARAM_PHONETIC_ENCODER = HapiExtensions.EXT_SEARCHPARAM_PHONETIC_ENCODER; 260 261 public static final String VALUESET_FILTER_DISPLAY = "display"; 262 263 /** 264 * The name of the default partition 265 */ 266 public static final String DEFAULT_PARTITION_NAME = ProviderConstants.DEFAULT_PARTITION_NAME; 267 268 /** 269 * The name of the collection of all partitions 270 */ 271 public static final String ALL_PARTITIONS_NAME = "ALL_PARTITIONS"; 272 273 /** 274 * Parameter for the $expand operation 275 */ 276 public static final String OPERATION_EXPAND_PARAM_INCLUDE_HIERARCHY = "includeHierarchy"; 277 278 public static final String OPERATION_EXPAND_PARAM_DISPLAY_LANGUAGE = "displayLanguage"; 279 public static final String HEADER_UPSERT_EXISTENCE_CHECK = "X-Upsert-Extistence-Check"; 280 public static final String HEADER_UPSERT_EXISTENCE_CHECK_DISABLED = "disabled"; 281 282 /** 283 * Parameters for the rewrite history operation 284 */ 285 public static final String HEADER_REWRITE_HISTORY = "X-Rewrite-History"; 286 287 public static final String SKIP_REINDEX_ON_UPDATE = "SKIP-REINDEX-ON-UPDATE"; 288 /** 289 * IPS Generation operation name 290 */ 291 public static final String OPERATION_SUMMARY = "$summary"; 292 /** 293 * IPS Generation operation URL 294 */ 295 public static final String SUMMARY_OPERATION_URL = "http://hl7.org/fhir/uv/ips/OperationDefinition/summary"; 296 297 public static final String BULK_META_EXTENSION_EXPORT_IDENTIFIER = 298 "https://hapifhir.org/NamingSystem/bulk-export-identifier"; 299 public static final String BULK_META_EXTENSION_JOB_ID = "https://hapifhir.org/NamingSystem/bulk-export-job-id"; 300 public static final String BULK_META_EXTENSION_RESOURCE_TYPE = 301 "https://hapifhir.org/NamingSystem/bulk-export-binary-resource-type"; 302 public static final Set<String> UNDESIRED_RESOURCE_LINKAGES_FOR_EVERYTHING_ON_PATIENT_INSTANCE = 303 Set.of("Provenance", "List", "Group"); 304 public static final String HAPI_DATABASE_PARTITION_MODE = "hapi.database_partition_mode"; 305 public static final String HAPI_DATABASE_PARTITION_MODE_DEFAULT = "false"; 306 307 /** 308 * Non-instantiable 309 */ 310 private JpaConstants() { 311 // nothing 312 } 313}