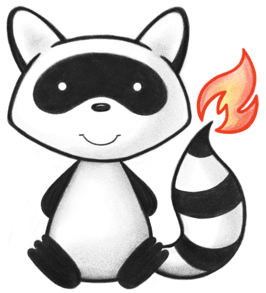
001/*- 002 * #%L 003 * HAPI FHIR JPA - Search Parameters 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.interceptor.model; 021 022import ca.uhn.fhir.jpa.searchparam.SearchParameterMap; 023import ca.uhn.fhir.rest.api.RestOperationTypeEnum; 024import jakarta.annotation.Nonnull; 025import jakarta.annotation.Nullable; 026import org.hl7.fhir.instance.model.api.IBaseResource; 027import org.hl7.fhir.instance.model.api.IIdType; 028 029import static org.apache.commons.lang3.StringUtils.isNotBlank; 030 031/** 032 * This is a model class used as a parameter for the interceptor pointcut 033 * {@link ca.uhn.fhir.interceptor.api.Pointcut#STORAGE_PARTITION_IDENTIFY_READ}. 034 */ 035public class ReadPartitionIdRequestDetails extends PartitionIdRequestDetails { 036 037 private final String myResourceType; 038 private final RestOperationTypeEnum myRestOperationType; 039 private final IIdType myReadResourceId; 040 041 @Nullable 042 private final SearchParameterMap mySearchParams; 043 044 @Nullable 045 private final IBaseResource myConditionalTargetOrNull; 046 047 @Nullable 048 private final String mySearchUuid; 049 050 @Nullable 051 private final String myExtendedOperationName; 052 053 private ReadPartitionIdRequestDetails( 054 String theResourceType, 055 RestOperationTypeEnum theRestOperationType, 056 IIdType theReadResourceId, 057 @Nullable SearchParameterMap theSearchParams, 058 @Nullable IBaseResource theConditionalTargetOrNull, 059 @Nullable String theSearchUuid, 060 String theExtendedOperationName) { 061 myResourceType = theResourceType; 062 myRestOperationType = theRestOperationType; 063 myReadResourceId = theReadResourceId; 064 mySearchParams = theSearchParams; 065 myConditionalTargetOrNull = theConditionalTargetOrNull; 066 mySearchUuid = theSearchUuid; 067 myExtendedOperationName = theExtendedOperationName; 068 } 069 070 @Nullable 071 public String getExtendedOperationName() { 072 return myExtendedOperationName; 073 } 074 075 @Nullable 076 public String getSearchUuid() { 077 return mySearchUuid; 078 } 079 080 public String getResourceType() { 081 return myResourceType; 082 } 083 084 public RestOperationTypeEnum getRestOperationType() { 085 return myRestOperationType; 086 } 087 088 public IIdType getReadResourceId() { 089 return myReadResourceId; 090 } 091 092 @Nullable 093 public SearchParameterMap getSearchParams() { 094 return mySearchParams; 095 } 096 097 @Nullable 098 public IBaseResource getConditionalTargetOrNull() { 099 return myConditionalTargetOrNull; 100 } 101 102 /** 103 * @param theId The resource ID (must include a resource type and ID) 104 */ 105 public static ReadPartitionIdRequestDetails forRead(IIdType theId) { 106 assert isNotBlank(theId.getResourceType()); 107 assert isNotBlank(theId.getIdPart()); 108 return forRead(theId.getResourceType(), theId, false); 109 } 110 111 public static ReadPartitionIdRequestDetails forServerOperation(@Nonnull String theOperationName) { 112 return new ReadPartitionIdRequestDetails( 113 null, RestOperationTypeEnum.EXTENDED_OPERATION_SERVER, null, null, null, null, theOperationName); 114 } 115 116 /** 117 * @since 7.4.0 118 */ 119 public static ReadPartitionIdRequestDetails forDelete(@Nonnull String theResourceType, @Nonnull IIdType theId) { 120 RestOperationTypeEnum op = RestOperationTypeEnum.DELETE; 121 return new ReadPartitionIdRequestDetails( 122 theResourceType, op, theId.withResourceType(theResourceType), null, null, null, null); 123 } 124 125 /** 126 * @since 7.4.0 127 */ 128 public static ReadPartitionIdRequestDetails forPatch(String theResourceType, IIdType theId) { 129 RestOperationTypeEnum op = RestOperationTypeEnum.PATCH; 130 return new ReadPartitionIdRequestDetails( 131 theResourceType, op, theId.withResourceType(theResourceType), null, null, null, null); 132 } 133 134 public static ReadPartitionIdRequestDetails forRead( 135 String theResourceType, @Nonnull IIdType theId, boolean theIsVread) { 136 RestOperationTypeEnum op = theIsVread ? RestOperationTypeEnum.VREAD : RestOperationTypeEnum.READ; 137 return new ReadPartitionIdRequestDetails( 138 theResourceType, op, theId.withResourceType(theResourceType), null, null, null, null); 139 } 140 141 public static ReadPartitionIdRequestDetails forSearchType( 142 String theResourceType, SearchParameterMap theParams, IBaseResource theConditionalOperationTargetOrNull) { 143 return new ReadPartitionIdRequestDetails( 144 theResourceType, 145 RestOperationTypeEnum.SEARCH_TYPE, 146 null, 147 theParams != null ? theParams : SearchParameterMap.newSynchronous(), 148 theConditionalOperationTargetOrNull, 149 null, 150 null); 151 } 152 153 public static ReadPartitionIdRequestDetails forHistory(String theResourceType, IIdType theIdType) { 154 RestOperationTypeEnum restOperationTypeEnum; 155 if (theIdType != null) { 156 restOperationTypeEnum = RestOperationTypeEnum.HISTORY_INSTANCE; 157 } else if (theResourceType != null) { 158 restOperationTypeEnum = RestOperationTypeEnum.HISTORY_TYPE; 159 } else { 160 restOperationTypeEnum = RestOperationTypeEnum.HISTORY_SYSTEM; 161 } 162 return new ReadPartitionIdRequestDetails( 163 theResourceType, restOperationTypeEnum, theIdType, null, null, null, null); 164 } 165 166 public static ReadPartitionIdRequestDetails forSearchUuid(String theUuid) { 167 return new ReadPartitionIdRequestDetails(null, RestOperationTypeEnum.GET_PAGE, null, null, null, theUuid, null); 168 } 169}