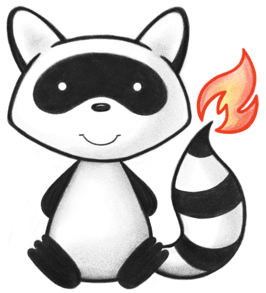
001/*- 002 * #%L 003 * HAPI FHIR JPA - Search Parameters 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.cache; 021 022import ca.uhn.fhir.jpa.searchparam.SearchParameterMap; 023 024import java.time.Instant; 025 026/** 027 * This is a handle to the cache created by {@link IResourceChangeListenerRegistry} when a listener is registered. 028 * This this handle can be used to refresh the cache if required. 029 */ 030public interface IResourceChangeListenerCache { 031 /** 032 * @return the search parameter map the listener was registered with 033 */ 034 SearchParameterMap getSearchParameterMap(); 035 036 /** 037 * @return whether the cache has been initialized. (If not, the cache will be empty.) 038 */ 039 boolean isInitialized(); 040 041 /** 042 * @return the name of the resource type the listener was registered with 043 */ 044 String getResourceName(); 045 046 /** 047 * @return the next scheduled time the cache will search the repository, update its cache and notify 048 * its listener of any changes 049 */ 050 Instant getNextRefreshTime(); 051 052 /** 053 * sets the nextRefreshTime to {@link Instant.MIN} so that the cache will be refreshed and listeners notified in another thread 054 * the next time cache refresh times are checked (every {@link ResourceChangeListenerCacheRefresherImpl.LOCAL_REFRESH_INTERVAL_MS}. 055 */ 056 void requestRefresh(); 057 058 /** 059 * Refresh the cache immediately in the current thread and notify its listener if there are any changes 060 * @return counts of detected resource creates, updates and deletes 061 */ 062 ResourceChangeResult forceRefresh(); 063 064 /** 065 * If nextRefreshTime is in the past, then update the cache with the current repository contents and notify its listener of any changes 066 * @return counts of detected resource creates, updates and deletes 067 */ 068 ResourceChangeResult refreshCacheIfNecessary(); 069 070 // TODO KHS in the future support adding new listeners to existing caches 071}