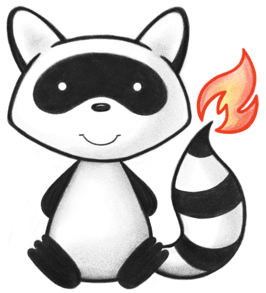
001/*- 002 * #%L 003 * HAPI FHIR JPA - Search Parameters 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.cache; 021 022import ca.uhn.fhir.jpa.searchparam.SearchParameterMap; 023import com.google.common.annotations.VisibleForTesting; 024import org.hl7.fhir.instance.model.api.IBaseResource; 025 026import java.util.Set; 027 028/** 029 * This component holds an in-memory list of all registered {@link IResourceChangeListener} instances along 030 * with their caches and other details needed to maintain those caches. Register an {@link IResourceChangeListener} instance 031 * with this service to be notified when resources you care about are changed. This service quickly notifies listeners 032 * of changes that happened on the local process and also eventually notifies listeners of changes that were made by 033 * remote processes. 034 */ 035public interface IResourceChangeListenerRegistry { 036 037 /** 038 * Register a listener in order to be notified whenever a resource matching the provided SearchParameterMap 039 * changes in any way. If the change happened on the same jvm process where this registry resides, then the listener will be called 040 * within {@link ResourceChangeListenerCacheRefresherImpl#LOCAL_REFRESH_INTERVAL_MS} of the change happening. If the change happened 041 * on a different jvm process, then the listener will be called within the time specified in theRemoteRefreshIntervalMs parameter. 042 * @param theResourceName the type of the resource the listener should be notified about (e.g. "Subscription" or "SearchParameter") 043 * @param theSearchParameterMap the listener will only be notified of changes to resources that match this map 044 * @param theResourceChangeListener the listener that will be called whenever resource changes are detected 045 * @param theRemoteRefreshIntervalMs the number of milliseconds between checking the database for changed resources that match the search parameter map 046 * @throws ca.uhn.fhir.parser.DataFormatException if theResourceName is not a valid resource type in the FhirContext 047 * @throws IllegalArgumentException if theSearchParamMap cannot be evaluated in-memory 048 * @return RegisteredResourceChangeListener a handle to the created cache that can be used to manually refresh the cache if required 049 */ 050 IResourceChangeListenerCache registerResourceResourceChangeListener( 051 String theResourceName, 052 SearchParameterMap theSearchParameterMap, 053 IResourceChangeListener theResourceChangeListener, 054 long theRemoteRefreshIntervalMs); 055 056 /** 057 * Unregister a listener from this service 058 * 059 * @param theResourceChangeListener 060 */ 061 void unregisterResourceResourceChangeListener(IResourceChangeListener theResourceChangeListener); 062 063 /** 064 * Unregister a listener from this service using its cache handle 065 * 066 * @param theResourceChangeListenerCache 067 */ 068 void unregisterResourceResourceChangeListener(IResourceChangeListenerCache theResourceChangeListenerCache); 069 070 @VisibleForTesting 071 void clearListenersForUnitTest(); 072 073 /** 074 * 075 * @param theCache 076 * @return true if theCache is registered 077 */ 078 boolean contains(IResourceChangeListenerCache theCache); 079 080 /** 081 * Called by the {@link ResourceChangeListenerRegistryInterceptor} when a resource is changed to invalidate matching 082 * caches so their listeners are notified the next time the caches are refreshed. 083 * @param theResource the resource that changed that might trigger a refresh 084 */ 085 void requestRefreshIfWatching(IBaseResource theResource); 086 087 /** 088 * @return a set of resource names watched by the registered listeners 089 */ 090 Set<String> getWatchedResourceNames(); 091}