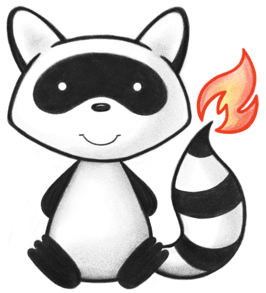
001/*- 002 * #%L 003 * HAPI FHIR JPA - Search Parameters 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.cache; 021 022import ca.uhn.fhir.model.primitive.IdDt; 023import org.apache.commons.lang3.builder.ToStringBuilder; 024import org.hl7.fhir.instance.model.api.IIdType; 025 026import java.util.ArrayList; 027import java.util.Collection; 028import java.util.Collections; 029import java.util.List; 030 031/** 032 * An immutable list of resource ids that have been changed, updated, or deleted. 033 */ 034public class ResourceChangeEvent implements IResourceChangeEvent { 035 private final List<IIdType> myCreatedResourceIds; 036 private final List<IIdType> myUpdatedResourceIds; 037 private final List<IIdType> myDeletedResourceIds; 038 039 private ResourceChangeEvent( 040 Collection<IIdType> theCreatedResourceIds, 041 Collection<IIdType> theUpdatedResourceIds, 042 Collection<IIdType> theDeletedResourceIds) { 043 myCreatedResourceIds = copyFrom(theCreatedResourceIds); 044 myUpdatedResourceIds = copyFrom(theUpdatedResourceIds); 045 myDeletedResourceIds = copyFrom(theDeletedResourceIds); 046 } 047 048 public static ResourceChangeEvent fromCreatedUpdatedDeletedResourceIds( 049 List<IIdType> theCreatedResourceIds, 050 List<IIdType> theUpdatedResourceIds, 051 List<IIdType> theDeletedResourceIds) { 052 return new ResourceChangeEvent(theCreatedResourceIds, theUpdatedResourceIds, theDeletedResourceIds); 053 } 054 055 private List<IIdType> copyFrom(Collection<IIdType> theResourceIds) { 056 ArrayList<IdDt> retval = new ArrayList<>(); 057 theResourceIds.forEach(id -> retval.add(new IdDt(id))); 058 return Collections.unmodifiableList(retval); 059 } 060 061 @Override 062 public List<IIdType> getCreatedResourceIds() { 063 return myCreatedResourceIds; 064 } 065 066 @Override 067 public List<IIdType> getUpdatedResourceIds() { 068 return myUpdatedResourceIds; 069 } 070 071 @Override 072 public List<IIdType> getDeletedResourceIds() { 073 return myDeletedResourceIds; 074 } 075 076 @Override 077 public boolean isEmpty() { 078 return myCreatedResourceIds.isEmpty() && myUpdatedResourceIds.isEmpty() && myDeletedResourceIds.isEmpty(); 079 } 080 081 @Override 082 public String toString() { 083 return new ToStringBuilder(this) 084 .append("myCreatedResourceIds", myCreatedResourceIds) 085 .append("myUpdatedResourceIds", myUpdatedResourceIds) 086 .append("myDeletedResourceIds", myDeletedResourceIds) 087 .toString(); 088 } 089}