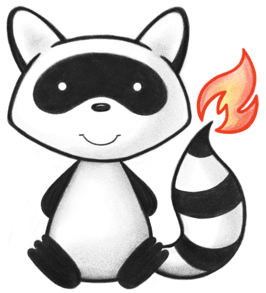
001/*- 002 * #%L 003 * HAPI FHIR JPA - Search Parameters 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.cache; 021 022import org.apache.commons.lang3.builder.ToStringBuilder; 023 024/** 025 * An immutable object containing the count of resource creates, updates and deletes detected by a cache refresh operation. 026 * Used internally for testing. 027 */ 028public class ResourceChangeResult { 029 public final long created; 030 public final long updated; 031 public final long deleted; 032 033 public ResourceChangeResult() { 034 created = 0; 035 updated = 0; 036 deleted = 0; 037 } 038 039 private ResourceChangeResult(long theCreated, long theUpdated, long theDeleted) { 040 created = theCreated; 041 updated = theUpdated; 042 deleted = theDeleted; 043 } 044 045 public static ResourceChangeResult fromCreated(int theCreated) { 046 return new ResourceChangeResult(theCreated, 0, 0); 047 } 048 049 public static ResourceChangeResult fromResourceChangeEvent(IResourceChangeEvent theResourceChangeEvent) { 050 return new ResourceChangeResult( 051 theResourceChangeEvent.getCreatedResourceIds().size(), 052 theResourceChangeEvent.getUpdatedResourceIds().size(), 053 theResourceChangeEvent.getDeletedResourceIds().size()); 054 } 055 056 public ResourceChangeResult plus(ResourceChangeResult theResult) { 057 return new ResourceChangeResult( 058 created + theResult.created, updated + theResult.updated, deleted + theResult.deleted); 059 } 060 061 @Override 062 public String toString() { 063 return new ToStringBuilder(this) 064 .append("created", created) 065 .append("updated", updated) 066 .append("deleted", deleted) 067 .toString(); 068 } 069}