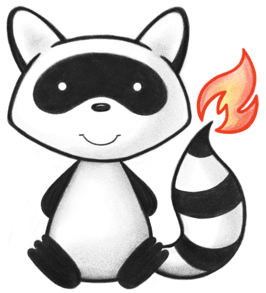
001/*- 002 * #%L 003 * HAPI FHIR JPA - Search Parameters 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.cache; 021 022import ca.uhn.fhir.model.primitive.IdDt; 023import org.hl7.fhir.instance.model.api.IIdType; 024 025import java.util.HashMap; 026import java.util.Map; 027import java.util.Set; 028 029/** 030 * This maintains a mapping of resource id to resource version. We cache these in order to 031 * detect resources that were modified on remote servers in our cluster. 032 */ 033public class ResourceVersionCache { 034 private final Map<IIdType, Long> myVersionMap = new HashMap<>(); 035 036 public void clear() { 037 myVersionMap.clear(); 038 } 039 040 /** 041 * @param theResourceId 042 * @param theVersion 043 * @return previous value 044 */ 045 public Long put(IIdType theResourceId, Long theVersion) { 046 return myVersionMap.put(new IdDt(theResourceId).toVersionless(), theVersion); 047 } 048 049 public Long getVersionForResourceId(IIdType theResourceId) { 050 return myVersionMap.get(new IdDt(theResourceId)); 051 } 052 053 public Long removeResourceId(IIdType theResourceId) { 054 return myVersionMap.remove(new IdDt(theResourceId)); 055 } 056 057 public void initialize(ResourceVersionMap theResourceVersionMap) { 058 for (IIdType resourceId : theResourceVersionMap.keySet()) { 059 myVersionMap.put(resourceId, theResourceVersionMap.get(resourceId)); 060 } 061 } 062 063 public int size() { 064 return myVersionMap.size(); 065 } 066 067 public Set<IIdType> keySet() { 068 return myVersionMap.keySet(); 069 } 070}