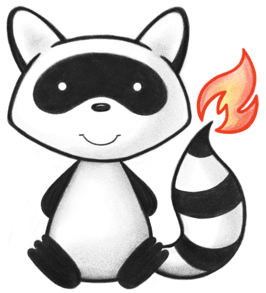
001/*- 002 * #%L 003 * HAPI FHIR JPA - Search Parameters 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.cache; 021 022import ca.uhn.fhir.jpa.model.cross.IBasePersistedResource; 023import ca.uhn.fhir.model.primitive.IdDt; 024import org.hl7.fhir.instance.model.api.IBaseResource; 025import org.hl7.fhir.instance.model.api.IIdType; 026import org.slf4j.Logger; 027import org.slf4j.LoggerFactory; 028 029import java.util.Collections; 030import java.util.HashMap; 031import java.util.HashSet; 032import java.util.List; 033import java.util.Map; 034import java.util.Set; 035 036/** 037 * This immutable map holds a copy of current resource versions read from the repository. 038 */ 039public class ResourceVersionMap { 040 private static final Logger ourLog = LoggerFactory.getLogger(ResourceVersionMap.class); 041 private final Set<IIdType> mySourceIds = new HashSet<>(); 042 // Key versionless id, value version 043 private final Map<IIdType, Long> myMap = new HashMap<>(); 044 045 private ResourceVersionMap() {} 046 047 public static ResourceVersionMap fromResourceTableEntities(List<? extends IBasePersistedResource<?>> theEntities) { 048 ResourceVersionMap retval = new ResourceVersionMap(); 049 theEntities.forEach(entity -> retval.add(entity.getIdDt())); 050 return retval; 051 } 052 053 public static ResourceVersionMap fromResources(List<? extends IBaseResource> theResources) { 054 ResourceVersionMap retval = new ResourceVersionMap(); 055 theResources.forEach(resource -> retval.add(resource.getIdElement())); 056 return retval; 057 } 058 059 public static ResourceVersionMap empty() { 060 return new ResourceVersionMap(); 061 } 062 063 public static ResourceVersionMap fromIdsWithVersions(List<IIdType> theFhirIds) { 064 ResourceVersionMap retval = new ResourceVersionMap(); 065 theFhirIds.forEach(retval::add); 066 return retval; 067 } 068 069 private void add(IIdType theId) { 070 if (theId.getVersionIdPart() == null) { 071 ourLog.warn("Not storing {} in ResourceVersionMap because it does not have a version.", theId); 072 return; 073 } 074 IdDt id = new IdDt(theId); 075 mySourceIds.add(id); 076 myMap.put(id.toUnqualifiedVersionless(), id.getVersionIdPartAsLong()); 077 } 078 079 public Long getVersion(IIdType theResourceId) { 080 return get(theResourceId); 081 } 082 083 public int size() { 084 return myMap.size(); 085 } 086 087 public Set<IIdType> keySet() { 088 return Collections.unmodifiableSet(myMap.keySet()); 089 } 090 091 public Set<IIdType> getSourceIds() { 092 return Collections.unmodifiableSet(mySourceIds); 093 } 094 095 public Long get(IIdType theId) { 096 return myMap.get(new IdDt(theId.toUnqualifiedVersionless())); 097 } 098 099 public boolean containsKey(IIdType theId) { 100 return myMap.containsKey(new IdDt(theId.toUnqualifiedVersionless())); 101 } 102 103 public boolean isEmpty() { 104 return myMap.isEmpty(); 105 } 106}