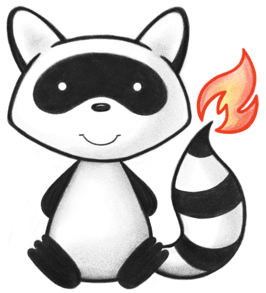
001/*- 002 * #%L 003 * HAPI FHIR JPA - Search Parameters 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.searchparam; 021 022import ca.uhn.fhir.model.api.IQueryParameterAnd; 023import ca.uhn.fhir.model.api.IQueryParameterType; 024import ca.uhn.fhir.rest.api.Constants; 025import ca.uhn.fhir.rest.param.HasAndListParam; 026import ca.uhn.fhir.rest.param.HasParam; 027import ca.uhn.fhir.rest.param.StringAndListParam; 028import ca.uhn.fhir.rest.param.StringParam; 029import ca.uhn.fhir.rest.param.TokenAndListParam; 030import ca.uhn.fhir.rest.param.TokenParam; 031import ca.uhn.fhir.rest.param.UriAndListParam; 032import ca.uhn.fhir.rest.param.UriParam; 033import org.hl7.fhir.instance.model.api.IAnyResource; 034 035import java.util.Collections; 036import java.util.HashMap; 037import java.util.Map; 038 039public class ResourceMetaParams { 040 /** 041 * These are parameters which are supported by searches 042 */ 043 public static final Map<String, Class<? extends IQueryParameterAnd<?>>> RESOURCE_META_AND_PARAMS; 044 /** 045 * These are parameters which are supported by searches 046 */ 047 public static final Map<String, Class<? extends IQueryParameterType>> RESOURCE_META_PARAMS; 048 049 static { 050 Map<String, Class<? extends IQueryParameterType>> resourceMetaParams = new HashMap<>(); 051 Map<String, Class<? extends IQueryParameterAnd<?>>> resourceMetaAndParams = new HashMap<>(); 052 resourceMetaParams.put(IAnyResource.SP_RES_ID, StringParam.class); 053 resourceMetaAndParams.put(IAnyResource.SP_RES_ID, StringAndListParam.class); 054 resourceMetaParams.put(Constants.PARAM_PID, TokenParam.class); 055 resourceMetaAndParams.put(Constants.PARAM_PID, TokenAndListParam.class); 056 resourceMetaParams.put(Constants.PARAM_TAG, TokenParam.class); 057 resourceMetaAndParams.put(Constants.PARAM_TAG, TokenAndListParam.class); 058 resourceMetaParams.put(Constants.PARAM_PROFILE, UriParam.class); 059 resourceMetaAndParams.put(Constants.PARAM_PROFILE, UriAndListParam.class); 060 resourceMetaParams.put(Constants.PARAM_SECURITY, TokenParam.class); 061 resourceMetaAndParams.put(Constants.PARAM_SECURITY, TokenAndListParam.class); 062 resourceMetaParams.put(Constants.PARAM_HAS, HasParam.class); 063 resourceMetaAndParams.put(Constants.PARAM_HAS, HasAndListParam.class); 064 RESOURCE_META_PARAMS = Collections.unmodifiableMap(resourceMetaParams); 065 RESOURCE_META_AND_PARAMS = Collections.unmodifiableMap(resourceMetaAndParams); 066 } 067}