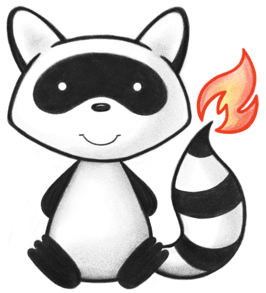
001/*- 002 * #%L 003 * HAPI FHIR JPA - Search Parameters 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.searchparam.extractor; 021 022import ca.uhn.fhir.interceptor.model.RequestPartitionId; 023import ca.uhn.fhir.rest.api.server.RequestDetails; 024import ca.uhn.fhir.rest.api.server.storage.TransactionDetails; 025import jakarta.annotation.Nonnull; 026 027public class CrossPartitionReferenceDetails { 028 029 @Nonnull 030 private final RequestPartitionId mySourceResourcePartitionId; 031 032 @Nonnull 033 private final PathAndRef myPathAndRef; 034 035 @Nonnull 036 private final RequestDetails myRequestDetails; 037 038 @Nonnull 039 private final TransactionDetails myTransactionDetails; 040 041 @Nonnull 042 private final String mySourceResourceName; 043 044 /** 045 * Constructor 046 */ 047 public CrossPartitionReferenceDetails( 048 @Nonnull RequestPartitionId theSourceResourcePartitionId, 049 @Nonnull String theSourceResourceName, 050 @Nonnull PathAndRef thePathAndRef, 051 @Nonnull RequestDetails theRequestDetails, 052 @Nonnull TransactionDetails theTransactionDetails) { 053 mySourceResourcePartitionId = theSourceResourcePartitionId; 054 mySourceResourceName = theSourceResourceName; 055 myPathAndRef = thePathAndRef; 056 myRequestDetails = theRequestDetails; 057 myTransactionDetails = theTransactionDetails; 058 } 059 060 @Nonnull 061 public String getSourceResourceName() { 062 return mySourceResourceName; 063 } 064 065 @Nonnull 066 public RequestDetails getRequestDetails() { 067 return myRequestDetails; 068 } 069 070 @Nonnull 071 public TransactionDetails getTransactionDetails() { 072 return myTransactionDetails; 073 } 074 075 @Nonnull 076 public RequestPartitionId getSourceResourcePartitionId() { 077 return mySourceResourcePartitionId; 078 } 079 080 @Nonnull 081 public PathAndRef getPathAndRef() { 082 return myPathAndRef; 083 } 084}