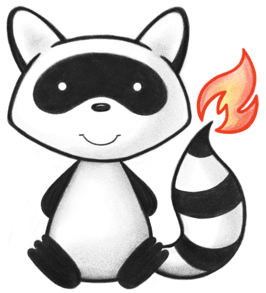
001/*- 002 * #%L 003 * HAPI FHIR JPA - Search Parameters 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.searchparam.extractor; 021 022import ca.uhn.fhir.interceptor.model.RequestPartitionId; 023import ca.uhn.fhir.jpa.model.cross.IResourceLookup; 024import ca.uhn.fhir.rest.api.server.RequestDetails; 025import ca.uhn.fhir.rest.api.server.storage.TransactionDetails; 026import jakarta.annotation.Nonnull; 027import jakarta.annotation.Nullable; 028import org.hl7.fhir.instance.model.api.IBaseResource; 029 030public interface IResourceLinkResolver { 031 032 /** 033 * This method resolves the target of a reference found within a resource that is being created/updated. We do this 034 * so that we can create indexed links between resources, and so that we can validate that the target actually 035 * exists in cases where we need to check that. 036 * <p> 037 * This method returns an {@link IResourceLookup} to avoid needing to resolve the entire resource. 038 * 039 * @param theRequestPartitionId The partition ID of the target resource 040 * @param theSourceResourceName The resource type for the resource containing the reference 041 * @param thePathAndRef The path and reference 042 * @param theRequest The incoming request, if any 043 * @param theTransactionDetails The current TransactionDetails object 044 */ 045 IResourceLookup findTargetResource( 046 @Nonnull RequestPartitionId theRequestPartitionId, 047 String theSourceResourceName, 048 PathAndRef thePathAndRef, 049 RequestDetails theRequest, 050 TransactionDetails theTransactionDetails); 051 052 /** 053 * This method resolves the target of a reference found within a resource that is being created/updated. We do this 054 * so that we can create indexed links between resources, and so that we can validate that the target actually 055 * exists in cases where we need to check that. 056 * <p> 057 * This method returns an {@link IResourceLookup} to avoid needing to resolve the entire resource. 058 * 059 * @param theRequestPartitionId The partition ID of the target resource 060 * @param theSourceResourceName The resource type for the resource containing the reference 061 * @param thePathAndRef The path and reference 062 * @param theRequest The incoming request, if any 063 * @param theTransactionDetails The current TransactionDetails object 064 */ 065 @Nullable 066 IBaseResource loadTargetResource( 067 @Nonnull RequestPartitionId theRequestPartitionId, 068 String theSourceResourceName, 069 PathAndRef thePathAndRef, 070 RequestDetails theRequest, 071 TransactionDetails theTransactionDetails); 072 073 void validateTypeOrThrowException(Class<? extends IBaseResource> theType); 074}