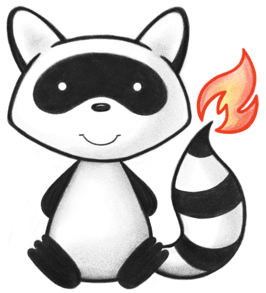
001/* 002 * #%L 003 * HAPI FHIR JPA - Search Parameters 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.searchparam.extractor; 021 022import ca.uhn.fhir.context.RuntimeSearchParam; 023import ca.uhn.fhir.jpa.model.entity.BaseResourceIndexedSearchParam; 024import ca.uhn.fhir.jpa.model.entity.ResourceIndexedComboStringUnique; 025import ca.uhn.fhir.jpa.model.entity.ResourceIndexedComboTokenNonUnique; 026import ca.uhn.fhir.jpa.model.entity.ResourceIndexedSearchParamDate; 027import ca.uhn.fhir.jpa.model.entity.ResourceIndexedSearchParamNumber; 028import ca.uhn.fhir.jpa.model.entity.ResourceIndexedSearchParamQuantity; 029import ca.uhn.fhir.jpa.model.entity.ResourceIndexedSearchParamQuantityNormalized; 030import ca.uhn.fhir.jpa.model.entity.ResourceIndexedSearchParamString; 031import ca.uhn.fhir.jpa.model.entity.ResourceIndexedSearchParamToken; 032import ca.uhn.fhir.jpa.model.entity.ResourceIndexedSearchParamUri; 033import org.hl7.fhir.instance.model.api.IBase; 034import org.hl7.fhir.instance.model.api.IBaseResource; 035 036import java.util.ArrayList; 037import java.util.Collection; 038import java.util.Collections; 039import java.util.Date; 040import java.util.HashSet; 041import java.util.List; 042 043public interface ISearchParamExtractor { 044 045 /** 046 * Constant for the {@literal theSearchParamFilter} parameters on this interface 047 * indicating that all search parameters should be extracted. 048 */ 049 ISearchParamFilter ALL_PARAMS = t -> t; 050 051 /** 052 * Constant for the {@literal theSearchParamFilter} parameters on this interface 053 * indicating that no search parameters should be extracted. 054 */ 055 ISearchParamFilter NO_PARAMS = t -> Collections.emptyList(); 056 057 default SearchParamSet<ResourceIndexedSearchParamDate> extractSearchParamDates(IBaseResource theResource) { 058 return extractSearchParamDates(theResource, ALL_PARAMS); 059 } 060 061 SearchParamSet<ResourceIndexedSearchParamDate> extractSearchParamDates( 062 IBaseResource theResource, ISearchParamFilter theSearchParamFilter); 063 064 default SearchParamSet<ResourceIndexedSearchParamNumber> extractSearchParamNumber(IBaseResource theResource) { 065 return extractSearchParamNumber(theResource, ALL_PARAMS); 066 } 067 068 SearchParamSet<ResourceIndexedSearchParamNumber> extractSearchParamNumber( 069 IBaseResource theResource, ISearchParamFilter theSearchParamFilter); 070 071 default SearchParamSet<ResourceIndexedSearchParamQuantity> extractSearchParamQuantity(IBaseResource theResource) { 072 return extractSearchParamQuantity(theResource, ALL_PARAMS); 073 } 074 075 SearchParamSet<ResourceIndexedSearchParamQuantity> extractSearchParamQuantity( 076 IBaseResource theResource, ISearchParamFilter theSearchParamFilter); 077 078 default SearchParamSet<ResourceIndexedSearchParamQuantityNormalized> extractSearchParamQuantityNormalized( 079 IBaseResource theResource) { 080 return extractSearchParamQuantityNormalized(theResource, ALL_PARAMS); 081 } 082 083 SearchParamSet<ResourceIndexedSearchParamQuantityNormalized> extractSearchParamQuantityNormalized( 084 IBaseResource theResource, ISearchParamFilter theSearchParamFilter); 085 086 default SearchParamSet<ResourceIndexedSearchParamString> extractSearchParamStrings(IBaseResource theResource) { 087 return extractSearchParamStrings(theResource, ALL_PARAMS); 088 } 089 090 SearchParamSet<ResourceIndexedSearchParamString> extractSearchParamStrings( 091 IBaseResource theResource, ISearchParamFilter theSearchParamFilter); 092 093 default SearchParamSet<ResourceIndexedSearchParamComposite> extractSearchParamComposites( 094 IBaseResource theResource) { 095 return extractSearchParamComposites(theResource, ALL_PARAMS); 096 } 097 098 SearchParamSet<ResourceIndexedSearchParamComposite> extractSearchParamComposites( 099 IBaseResource theResource, ISearchParamFilter theSearchParamFilter); 100 101 default SearchParamSet<BaseResourceIndexedSearchParam> extractSearchParamTokens(IBaseResource theResource) { 102 return extractSearchParamTokens(theResource, ALL_PARAMS); 103 } 104 105 SearchParamSet<BaseResourceIndexedSearchParam> extractSearchParamTokens( 106 IBaseResource theResource, ISearchParamFilter theSearchParamFilter); 107 108 SearchParamSet<BaseResourceIndexedSearchParam> extractSearchParamTokens( 109 IBaseResource theResource, RuntimeSearchParam theSearchParam); 110 111 SearchParamSet<BaseResourceIndexedSearchParam> extractSearchParamSpecial( 112 IBaseResource theResource, ISearchParamFilter theSearchParamFilter); 113 114 SearchParamSet<ResourceIndexedComboStringUnique> extractSearchParamComboUnique( 115 String theResourceType, ResourceIndexedSearchParams theParams); 116 117 SearchParamSet<ResourceIndexedComboTokenNonUnique> extractSearchParamComboNonUnique( 118 String theResourceType, ResourceIndexedSearchParams theParams); 119 120 default SearchParamSet<ResourceIndexedSearchParamUri> extractSearchParamUri(IBaseResource theResource) { 121 return extractSearchParamUri(theResource, ALL_PARAMS); 122 } 123 124 SearchParamSet<ResourceIndexedSearchParamUri> extractSearchParamUri( 125 IBaseResource theResource, ISearchParamFilter theSearchParamFilter); 126 127 SearchParamSet<PathAndRef> extractResourceLinks(IBaseResource theResource, boolean theWantLocalReferences); 128 129 String[] split(String theExpression); 130 131 List<String> extractParamValuesAsStrings(RuntimeSearchParam theActiveSearchParam, IBaseResource theResource); 132 133 List<IBase> extractValues(String thePaths, IBase theResource); 134 135 String toRootTypeName(IBase nextObject); 136 137 String toTypeName(IBase nextObject); 138 139 PathAndRef extractReferenceLinkFromResource(IBase theValue, String thePath); 140 141 Date extractDateFromResource(IBase theValue, String thePath); 142 143 ResourceIndexedSearchParamToken createSearchParamForCoding( 144 String theResourceType, RuntimeSearchParam theSearchParam, IBase theValue); 145 146 String getDisplayTextForCoding(IBase theValue); 147 148 BaseSearchParamExtractor.IValueExtractor getPathValueExtractor(IBase theResource, String theSinglePath); 149 150 List<IBase> getCodingsFromCodeableConcept(IBase theValue); 151 152 String getDisplayTextFromCodeableConcept(IBase theValue); 153 154 @FunctionalInterface 155 interface ISearchParamFilter { 156 157 /** 158 * Given the list of search parameters for extracting, an implementation of this 159 * interface may selectively remove any that it wants to remove (or can add if desired). 160 * <p> 161 * Implementations must not modify the list that is passed in. If changes are 162 * desired, a new list must be created and returned. 163 */ 164 Collection<RuntimeSearchParam> filterSearchParams(Collection<RuntimeSearchParam> theSearchParams); 165 } 166 167 class SearchParamSet<T> extends HashSet<T> { 168 169 private List<String> myWarnings; 170 171 public void addWarning(String theWarning) { 172 if (myWarnings == null) { 173 myWarnings = new ArrayList<>(); 174 } 175 myWarnings.add(theWarning); 176 } 177 178 List<String> getWarnings() { 179 if (myWarnings == null) { 180 return Collections.emptyList(); 181 } 182 return myWarnings; 183 } 184 } 185}