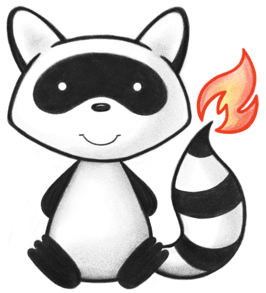
001/*- 002 * #%L 003 * HAPI FHIR JPA - Search Parameters 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.searchparam.extractor; 021 022import ca.uhn.fhir.jpa.model.entity.StorageSettings; 023import org.hl7.fhir.instance.model.api.IIdType; 024 025import java.util.Set; 026 027import static org.apache.commons.lang3.StringUtils.trim; 028 029public class LogicalReferenceHelper { 030 031 public static boolean isLogicalReference(StorageSettings myConfig, IIdType theId) { 032 Set<String> treatReferencesAsLogical = myConfig.getTreatReferencesAsLogical(); 033 if (treatReferencesAsLogical != null) { 034 for (String nextLogicalRef : treatReferencesAsLogical) { 035 nextLogicalRef = trim(nextLogicalRef); 036 if (nextLogicalRef.charAt(nextLogicalRef.length() - 1) == '*') { 037 if (theId.getValue().startsWith(nextLogicalRef.substring(0, nextLogicalRef.length() - 1))) { 038 return true; 039 } 040 } else { 041 if (theId.getValue().equals(nextLogicalRef)) { 042 return true; 043 } 044 } 045 } 046 } 047 048 /* 049 * Account for common logical references 050 */ 051 052 if (theId.getValue().startsWith("http://fhir.org/guides/argonaut/")) { 053 return true; 054 } 055 056 return false; 057 } 058}