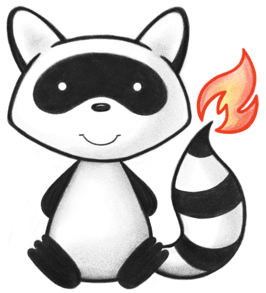
001/* 002 * #%L 003 * HAPI FHIR JPA - Search Parameters 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.searchparam.extractor; 021 022import org.apache.commons.lang3.builder.ToStringBuilder; 023import org.apache.commons.lang3.builder.ToStringStyle; 024import org.hl7.fhir.instance.model.api.IBaseReference; 025import org.hl7.fhir.instance.model.api.IBaseResource; 026 027public class PathAndRef { 028 029 private final String myPath; 030 private final IBaseReference myRef; 031 private final IBaseResource myResource; 032 private final String mySearchParamName; 033 private final boolean myCanonical; 034 035 /** 036 * Constructor for a reference 037 */ 038 public PathAndRef(String theSearchParamName, String thePath, IBaseReference theRef, boolean theCanonical) { 039 super(); 040 mySearchParamName = theSearchParamName; 041 myPath = thePath; 042 myRef = theRef; 043 myCanonical = theCanonical; 044 myResource = null; 045 } 046 047 /** 048 * Constructor for a resource (this is expected to be rare, only really covering 049 * cases like the path Bundle.entry.resource) 050 */ 051 public PathAndRef(String theSearchParamName, String thePath, IBaseResource theResource) { 052 super(); 053 mySearchParamName = theSearchParamName; 054 myPath = thePath; 055 myRef = null; 056 myCanonical = false; 057 myResource = theResource; 058 } 059 060 /** 061 * Note that this will generally be null, it is only used for cases like 062 * indexing {@literal Bundle.entry.resource}. If this is populated, {@link #getRef()} 063 * will be null and vice versa. 064 * 065 * @since 6.6.0 066 */ 067 public IBaseResource getResource() { 068 return myResource; 069 } 070 071 public boolean isCanonical() { 072 return myCanonical; 073 } 074 075 public String getSearchParamName() { 076 return mySearchParamName; 077 } 078 079 public String getPath() { 080 return myPath; 081 } 082 083 /** 084 * If this is populated, {@link #getResource()} will be null, and vice versa. 085 */ 086 public IBaseReference getRef() { 087 return myRef; 088 } 089 090 @Override 091 public String toString() { 092 ToStringBuilder b = new ToStringBuilder(this, ToStringStyle.SHORT_PREFIX_STYLE); 093 b.append("paramName", mySearchParamName); 094 if (myRef != null && myRef.getReferenceElement() != null) { 095 b.append("ref", myRef.getReferenceElement().getValue()); 096 } 097 b.append("path", myPath); 098 b.append("resource", myResource); 099 b.append("canonical", myCanonical); 100 return b.toString(); 101 } 102}