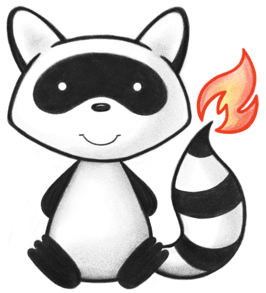
001/*- 002 * #%L 003 * HAPI FHIR JPA - Search Parameters 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.searchparam.extractor; 021 022import ca.uhn.fhir.context.RuntimeSearchParam; 023import ca.uhn.fhir.jpa.model.entity.BaseResourceIndexedSearchParam; 024import ca.uhn.fhir.rest.api.RestSearchParameterTypeEnum; 025import org.apache.commons.lang3.builder.ToStringBuilder; 026import org.apache.commons.lang3.builder.ToStringStyle; 027 028import java.util.ArrayList; 029import java.util.List; 030 031/** 032 * Intermediate holder for indexing composite search parameters. 033 * There will be one instance for each element extracted by the parent composite path expression. 034 */ 035public class ResourceIndexedSearchParamComposite { 036 037 private final String mySearchParamName; 038 private final String myPath; 039 private final List<Component> myComponents = new ArrayList<>(); 040 041 public ResourceIndexedSearchParamComposite(String theSearchParamName, String thePath) { 042 mySearchParamName = theSearchParamName; 043 myPath = thePath; 044 } 045 046 /** 047 * the SP name for this composite SP 048 */ 049 public String getSearchParamName() { 050 return mySearchParamName; 051 } 052 053 /** 054 * The path expression of the composite SP 055 */ 056 public String getPath() { 057 return myPath; 058 } 059 060 /** 061 * Subcomponent index data for this composite 062 */ 063 public List<Component> getComponents() { 064 return myComponents; 065 } 066 067 @Override 068 public String toString() { 069 return ToStringBuilder.reflectionToString(this, ToStringStyle.SHORT_PREFIX_STYLE); 070 } 071 072 /** 073 * Add subcomponent index data. 074 * @param theComponentSearchParam the component SP we are extracting 075 * @param theExtractedParams index data extracted by the sub-extractor 076 */ 077 public void addComponentIndexedSearchParams( 078 RuntimeSearchParam theComponentSearchParam, 079 ISearchParamExtractor.SearchParamSet<BaseResourceIndexedSearchParam> theExtractedParams) { 080 addComponentIndexedSearchParams( 081 theComponentSearchParam.getName(), theComponentSearchParam.getParamType(), theExtractedParams); 082 } 083 084 public void addComponentIndexedSearchParams( 085 String theComponentSearchParamName, 086 RestSearchParameterTypeEnum theComponentSearchParamType, 087 ISearchParamExtractor.SearchParamSet<BaseResourceIndexedSearchParam> theExtractedParams) { 088 myComponents.add(new Component(theComponentSearchParamName, theComponentSearchParamType, theExtractedParams)); 089 } 090 091 /** 092 * Nested holder of index data for a single component of a composite SP. 093 * E.g. hold token info for component-code under parent of component-code-value-quantity. 094 */ 095 public static class Component { 096 /** 097 * The SP name of this subcomponent. 098 * E.g. "component-code" when the parent composite SP is component-code-value-quantity. 099 */ 100 private final String mySearchParamName; 101 /** 102 * The SP type of this subcomponent. 103 * E.g. TOKEN when indexing "component-code" of parent composite SP is component-code-value-quantity. 104 */ 105 private final RestSearchParameterTypeEnum mySearchParameterType; 106 /** 107 * Any of the extracted data of any type for this subcomponent. 108 */ 109 private final ISearchParamExtractor.SearchParamSet<BaseResourceIndexedSearchParam> myParamIndexValues; 110 111 private Component( 112 String theComponentSearchParamName, 113 RestSearchParameterTypeEnum theComponentSearchParamType, 114 ISearchParamExtractor.SearchParamSet<BaseResourceIndexedSearchParam> theParamIndexValues) { 115 mySearchParamName = theComponentSearchParamName; 116 mySearchParameterType = theComponentSearchParamType; 117 myParamIndexValues = theParamIndexValues; 118 } 119 120 public String getSearchParamName() { 121 return mySearchParamName; 122 } 123 124 public RestSearchParameterTypeEnum getSearchParameterType() { 125 return mySearchParameterType; 126 } 127 128 public ISearchParamExtractor.SearchParamSet<BaseResourceIndexedSearchParam> getParamIndexValues() { 129 return myParamIndexValues; 130 } 131 132 @Override 133 public String toString() { 134 return ToStringBuilder.reflectionToString(this, ToStringStyle.SHORT_PREFIX_STYLE); 135 } 136 } 137}