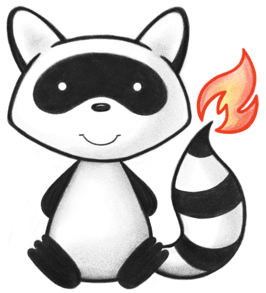
001/* 002 * #%L 003 * HAPI FHIR JPA - Search Parameters 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.searchparam.extractor; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.jpa.model.config.PartitionSettings; 024import ca.uhn.fhir.jpa.model.entity.StorageSettings; 025import ca.uhn.fhir.model.dstu2.composite.ContactPointDt; 026import ca.uhn.fhir.rest.server.util.ISearchParamRegistry; 027import ca.uhn.fhir.util.FhirTerser; 028import org.hl7.fhir.instance.model.api.IBase; 029import org.hl7.fhir.instance.model.api.IBaseDatatype; 030import org.hl7.fhir.instance.model.api.IBaseExtension; 031 032import java.util.ArrayList; 033import java.util.List; 034 035public class SearchParamExtractorDstu2 extends BaseSearchParamExtractor implements ISearchParamExtractor { 036 037 public SearchParamExtractorDstu2() {} 038 039 /** 040 * Constructor for unit tests 041 */ 042 SearchParamExtractorDstu2( 043 StorageSettings theStorageSettings, 044 PartitionSettings thePartitionSettings, 045 FhirContext theCtx, 046 ISearchParamRegistry theSearchParamRegistry) { 047 super(theStorageSettings, thePartitionSettings, theCtx, theSearchParamRegistry); 048 start(); 049 } 050 051 @Override 052 public IValueExtractor getPathValueExtractor(IBase theResource, String theSinglePath) { 053 return () -> { 054 String path = theSinglePath; 055 056 String needContactPointSystem = null; 057 if (path.endsWith("(system=phone)")) { 058 path = path.substring(0, path.length() - "(system=phone)".length()); 059 needContactPointSystem = "phone"; 060 } 061 if (path.endsWith("(system=email)")) { 062 path = path.substring(0, path.length() - "(system=email)".length()); 063 needContactPointSystem = "email"; 064 } 065 066 List<IBase> values = new ArrayList<>(); 067 FhirTerser t = getContext().newTerser(); 068 List<IBase> allValues = t.getValues(theResource, path); 069 for (IBase next : allValues) { 070 if (next instanceof IBaseExtension) { 071 IBaseDatatype value = ((IBaseExtension) next).getValue(); 072 if (value != null) { 073 values.add(value); 074 } 075 } else { 076 077 if (needContactPointSystem != null) { 078 if (next instanceof ContactPointDt) { 079 if (!needContactPointSystem.equals(((ContactPointDt) next).getSystem())) { 080 continue; 081 } 082 } 083 } 084 085 values.add(next); 086 } 087 } 088 return values; 089 }; 090 } 091}