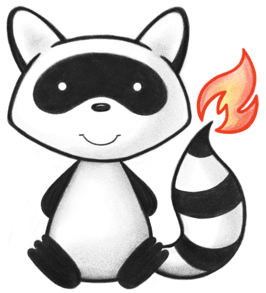
001/* 002 * #%L 003 * HAPI FHIR JPA - Search Parameters 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.searchparam.extractor; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.jpa.model.config.PartitionSettings; 024import ca.uhn.fhir.jpa.model.entity.StorageSettings; 025import ca.uhn.fhir.rest.server.util.ISearchParamRegistry; 026import com.google.common.annotations.VisibleForTesting; 027import jakarta.annotation.PostConstruct; 028import org.hl7.fhir.dstu3.context.IWorkerContext; 029import org.hl7.fhir.dstu3.fhirpath.FHIRPathEngine; 030import org.hl7.fhir.dstu3.hapi.ctx.HapiWorkerContext; 031import org.hl7.fhir.dstu3.model.Base; 032import org.hl7.fhir.instance.model.api.IBase; 033 034import java.util.ArrayList; 035import java.util.List; 036 037public class SearchParamExtractorDstu3 extends BaseSearchParamExtractor implements ISearchParamExtractor { 038 039 private FHIRPathEngine myFhirPathEngine; 040 041 /** 042 * Constructor 043 */ 044 public SearchParamExtractorDstu3() { 045 super(); 046 } 047 048 // This constructor is used by tests 049 @VisibleForTesting 050 public SearchParamExtractorDstu3( 051 StorageSettings theStorageSettings, 052 PartitionSettings thePartitionSettings, 053 FhirContext theCtx, 054 ISearchParamRegistry theSearchParamRegistry) { 055 super(theStorageSettings, thePartitionSettings, theCtx, theSearchParamRegistry); 056 initFhirPathEngine(); 057 start(); 058 } 059 060 @Override 061 public IValueExtractor getPathValueExtractor(IBase theResource, String theSinglePath) { 062 return () -> { 063 List<IBase> values = new ArrayList<>(); 064 List<Base> allValues = myFhirPathEngine.evaluate((Base) theResource, theSinglePath); 065 if (allValues.isEmpty() == false) { 066 values.addAll(allValues); 067 } 068 069 return values; 070 }; 071 } 072 073 @Override 074 @PostConstruct 075 public void start() { 076 super.start(); 077 if (myFhirPathEngine == null) { 078 initFhirPathEngine(); 079 } 080 } 081 082 public void initFhirPathEngine() { 083 IWorkerContext worker = new HapiWorkerContext(getContext(), getContext().getValidationSupport()); 084 myFhirPathEngine = new FHIRPathEngine(worker); 085 } 086}