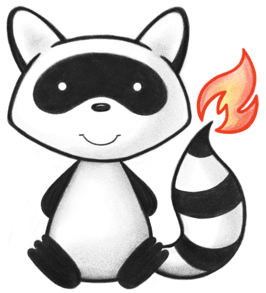
001/*- 002 * #%L 003 * HAPI FHIR JPA - Search Parameters 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.searchparam.matcher; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.jpa.model.entity.ResourceTable; 024import ca.uhn.fhir.jpa.searchparam.extractor.ISearchParamExtractor; 025import ca.uhn.fhir.jpa.searchparam.extractor.ResourceIndexedSearchParams; 026import ca.uhn.fhir.jpa.searchparam.extractor.SearchParamExtractorService; 027import ca.uhn.fhir.rest.api.server.RequestDetails; 028import ca.uhn.fhir.rest.api.server.storage.TransactionDetails; 029import jakarta.annotation.Nonnull; 030import org.hl7.fhir.instance.model.api.IBaseResource; 031import org.springframework.beans.factory.annotation.Autowired; 032 033public class IndexedSearchParamExtractor { 034 @Autowired 035 private FhirContext myContext; 036 037 @Autowired 038 private SearchParamExtractorService mySearchParamExtractorService; 039 040 @Nonnull 041 public ResourceIndexedSearchParams extractIndexedSearchParams( 042 IBaseResource theResource, RequestDetails theRequest) { 043 return extractIndexedSearchParams(theResource, theRequest, ISearchParamExtractor.ALL_PARAMS); 044 } 045 046 @Nonnull 047 public ResourceIndexedSearchParams extractIndexedSearchParams( 048 IBaseResource theResource, RequestDetails theRequest, ISearchParamExtractor.ISearchParamFilter filter) { 049 ResourceTable entity = new ResourceTable(); 050 TransactionDetails transactionDetails = new TransactionDetails(); 051 String resourceType = myContext.getResourceType(theResource); 052 entity.setResourceType(resourceType); 053 ResourceIndexedSearchParams resourceIndexedSearchParams = ResourceIndexedSearchParams.withSets(); 054 mySearchParamExtractorService.extractFromResource( 055 null, 056 theRequest, 057 resourceIndexedSearchParams, 058 ResourceIndexedSearchParams.empty(), 059 entity, 060 theResource, 061 transactionDetails, 062 false, 063 filter); 064 return resourceIndexedSearchParams; 065 } 066}