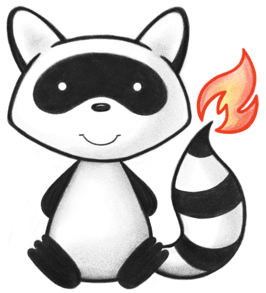
001/*- 002 * #%L 003 * HAPI FHIR JPA - Search Parameters 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.searchparam.retry; 021 022import ca.uhn.fhir.system.HapiSystemProperties; 023import org.apache.commons.lang3.Validate; 024import org.apache.commons.lang3.time.DateUtils; 025import org.slf4j.Logger; 026import org.slf4j.LoggerFactory; 027import org.springframework.beans.factory.BeanCreationException; 028import org.springframework.retry.RetryCallback; 029import org.springframework.retry.RetryContext; 030import org.springframework.retry.RetryListener; 031import org.springframework.retry.backoff.ExponentialBackOffPolicy; 032import org.springframework.retry.listener.RetryListenerSupport; 033import org.springframework.retry.policy.SimpleRetryPolicy; 034import org.springframework.retry.support.RetryTemplate; 035 036import java.util.function.Supplier; 037 038public class Retrier<T> { 039 private static final Logger ourLog = LoggerFactory.getLogger(Retrier.class); 040 041 private final Supplier<T> mySupplier; 042 043 private final RetryTemplate myRetryTemplate; 044 045 public Retrier(Supplier<T> theSupplier, int theMaxRetries) { 046 Validate.isTrue(theMaxRetries > 0, "maxRetries must be above zero."); 047 mySupplier = theSupplier; 048 049 myRetryTemplate = new RetryTemplate(); 050 051 ExponentialBackOffPolicy backOff = new ExponentialBackOffPolicy(); 052 backOff.setInitialInterval(500); 053 backOff.setMaxInterval(DateUtils.MILLIS_PER_MINUTE); 054 backOff.setMultiplier(2); 055 myRetryTemplate.setBackOffPolicy(backOff); 056 057 SimpleRetryPolicy retryPolicy = new SimpleRetryPolicy() { 058 private static final long serialVersionUID = -4522467251787518700L; 059 060 @Override 061 public boolean canRetry(RetryContext context) { 062 Throwable lastThrowable = context.getLastThrowable(); 063 if (lastThrowable instanceof BeanCreationException || lastThrowable instanceof NullPointerException) { 064 return false; 065 } 066 return super.canRetry(context); 067 } 068 }; 069 retryPolicy.setMaxAttempts(theMaxRetries); 070 myRetryTemplate.setRetryPolicy(retryPolicy); 071 072 RetryListener listener = new RetryListenerSupport() { 073 @Override 074 public <T, E extends Throwable> void onError( 075 RetryContext context, RetryCallback<T, E> callback, Throwable throwable) { 076 super.onError(context, callback, throwable); 077 if (throwable instanceof NullPointerException 078 || throwable instanceof UnsupportedOperationException 079 || HapiSystemProperties.isUnitTestModeEnabled()) { 080 ourLog.error( 081 "Retry failure {}/{}: {}", 082 context.getRetryCount(), 083 theMaxRetries, 084 throwable.getMessage(), 085 throwable); 086 } else { 087 ourLog.error( 088 "Retry failure {}/{}: {}", context.getRetryCount(), theMaxRetries, throwable.toString()); 089 } 090 } 091 }; 092 myRetryTemplate.registerListener(listener); 093 } 094 095 public T runWithRetry() { 096 return myRetryTemplate.execute(retryContext -> mySupplier.get()); 097 } 098}