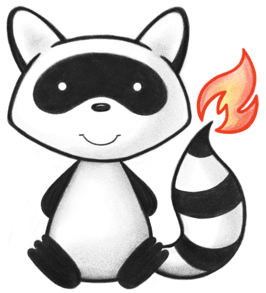
001/*- 002 * #%L 003 * HAPI FHIR JPA - Search Parameters 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.searchparam.util; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.context.FhirVersionEnum; 024import ca.uhn.fhir.i18n.Msg; 025import ca.uhn.fhir.rest.server.exceptions.InvalidRequestException; 026 027public class LastNParameterHelper { 028 029 public static boolean isLastNParameter(String theParamName, FhirContext theContext) { 030 if (theParamName == null) { 031 return false; 032 } 033 034 FhirVersionEnum version = theContext.getVersion().getVersion(); 035 036 if (isR5(version) && isLastNParameterR5(theParamName)) { 037 return true; 038 } else if (isR4(version) && isLastNParameterR4(theParamName)) { 039 return true; 040 } else if (isDstu3(version) && isLastNParameterDstu3(theParamName)) { 041 return true; 042 } else { 043 return false; 044 } 045 } 046 047 private static boolean isDstu3(FhirVersionEnum theVersion) { 048 return (theVersion == FhirVersionEnum.DSTU3); 049 } 050 051 private static boolean isR4(FhirVersionEnum theVersion) { 052 return (theVersion == FhirVersionEnum.R4); 053 } 054 055 private static boolean isR5(FhirVersionEnum theVersion) { 056 return (theVersion == FhirVersionEnum.R5); 057 } 058 059 private static boolean isLastNParameterDstu3(String theParamName) { 060 return (theParamName.equals(org.hl7.fhir.dstu3.model.Observation.SP_SUBJECT) 061 || theParamName.equals(org.hl7.fhir.dstu3.model.Observation.SP_PATIENT) 062 || theParamName.equals(org.hl7.fhir.dstu3.model.Observation.SP_CATEGORY) 063 || theParamName.equals(org.hl7.fhir.r4.model.Observation.SP_CODE)) 064 || theParamName.equals(org.hl7.fhir.dstu3.model.Observation.SP_DATE); 065 } 066 067 private static boolean isLastNParameterR4(String theParamName) { 068 return (theParamName.equals(org.hl7.fhir.r4.model.Observation.SP_SUBJECT) 069 || theParamName.equals(org.hl7.fhir.r4.model.Observation.SP_PATIENT) 070 || theParamName.equals(org.hl7.fhir.r4.model.Observation.SP_CATEGORY) 071 || theParamName.equals(org.hl7.fhir.r4.model.Observation.SP_CODE)) 072 || theParamName.equals(org.hl7.fhir.r4.model.Observation.SP_DATE); 073 } 074 075 private static boolean isLastNParameterR5(String theParamName) { 076 return (theParamName.equals(org.hl7.fhir.r4.model.Observation.SP_SUBJECT) 077 || theParamName.equals(org.hl7.fhir.r4.model.Observation.SP_PATIENT) 078 || theParamName.equals(org.hl7.fhir.r4.model.Observation.SP_CATEGORY) 079 || theParamName.equals(org.hl7.fhir.r4.model.Observation.SP_CODE)) 080 || theParamName.equals(org.hl7.fhir.r4.model.Observation.SP_DATE); 081 } 082 083 public static String getSubjectParamName(FhirContext theContext) { 084 if (theContext.getVersion().getVersion() == FhirVersionEnum.R5) { 085 return org.hl7.fhir.r4.model.Observation.SP_SUBJECT; 086 } else if (theContext.getVersion().getVersion() == FhirVersionEnum.R4) { 087 return org.hl7.fhir.r4.model.Observation.SP_SUBJECT; 088 } else if (theContext.getVersion().getVersion() == FhirVersionEnum.DSTU3) { 089 return org.hl7.fhir.dstu3.model.Observation.SP_SUBJECT; 090 } else { 091 throw new InvalidRequestException(Msg.code(489) + "$lastn operation is not implemented for FHIR Version " 092 + theContext.getVersion().getVersion().getFhirVersionString()); 093 } 094 } 095 096 public static String getPatientParamName(FhirContext theContext) { 097 if (theContext.getVersion().getVersion() == FhirVersionEnum.R5) { 098 return org.hl7.fhir.r4.model.Observation.SP_PATIENT; 099 } else if (theContext.getVersion().getVersion() == FhirVersionEnum.R4) { 100 return org.hl7.fhir.r4.model.Observation.SP_PATIENT; 101 } else if (theContext.getVersion().getVersion() == FhirVersionEnum.DSTU3) { 102 return org.hl7.fhir.dstu3.model.Observation.SP_PATIENT; 103 } else { 104 throw new InvalidRequestException(Msg.code(490) + "$lastn operation is not implemented for FHIR Version " 105 + theContext.getVersion().getVersion().getFhirVersionString()); 106 } 107 } 108 109 public static String getEffectiveParamName(FhirContext theContext) { 110 if (theContext.getVersion().getVersion() == FhirVersionEnum.R5) { 111 return org.hl7.fhir.r4.model.Observation.SP_DATE; 112 } else if (theContext.getVersion().getVersion() == FhirVersionEnum.R4) { 113 return org.hl7.fhir.r4.model.Observation.SP_DATE; 114 } else if (theContext.getVersion().getVersion() == FhirVersionEnum.DSTU3) { 115 return org.hl7.fhir.dstu3.model.Observation.SP_DATE; 116 } else { 117 throw new InvalidRequestException(Msg.code(491) + "$lastn operation is not implemented for FHIR Version " 118 + theContext.getVersion().getVersion().getFhirVersionString()); 119 } 120 } 121 122 public static String getCategoryParamName(FhirContext theContext) { 123 if (theContext.getVersion().getVersion() == FhirVersionEnum.R5) { 124 return org.hl7.fhir.r4.model.Observation.SP_CATEGORY; 125 } else if (theContext.getVersion().getVersion() == FhirVersionEnum.R4) { 126 return org.hl7.fhir.r4.model.Observation.SP_CATEGORY; 127 } else if (theContext.getVersion().getVersion() == FhirVersionEnum.DSTU3) { 128 return org.hl7.fhir.dstu3.model.Observation.SP_CATEGORY; 129 } else { 130 throw new InvalidRequestException(Msg.code(492) + "$lastn operation is not implemented for FHIR Version " 131 + theContext.getVersion().getVersion().getFhirVersionString()); 132 } 133 } 134 135 public static String getCodeParamName(FhirContext theContext) { 136 if (theContext.getVersion().getVersion() == FhirVersionEnum.R5) { 137 return org.hl7.fhir.r4.model.Observation.SP_CODE; 138 } else if (theContext.getVersion().getVersion() == FhirVersionEnum.R4) { 139 return org.hl7.fhir.r4.model.Observation.SP_CODE; 140 } else if (theContext.getVersion().getVersion() == FhirVersionEnum.DSTU3) { 141 return org.hl7.fhir.dstu3.model.Observation.SP_CODE; 142 } else { 143 throw new InvalidRequestException(Msg.code(493) + "$lastn operation is not implemented for FHIR Version " 144 + theContext.getVersion().getVersion().getFhirVersionString()); 145 } 146 } 147}