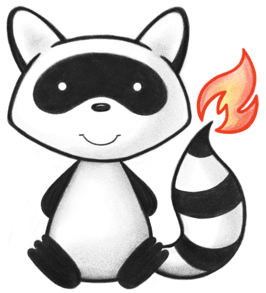
001package ca.uhn.fhir.jpa.subscription.async; 002 003/*- 004 * #%L 005 * HAPI FHIR Subscription Server 006 * %% 007 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 008 * %% 009 * Licensed under the Apache License, Version 2.0 (the "License"); 010 * you may not use this file except in compliance with the License. 011 * You may obtain a copy of the License at 012 * 013 * http://www.apache.org/licenses/LICENSE-2.0 014 * 015 * Unless required by applicable law or agreed to in writing, software 016 * distributed under the License is distributed on an "AS IS" BASIS, 017 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 018 * See the License for the specific language governing permissions and 019 * limitations under the License. 020 * #L% 021 */ 022 023import ca.uhn.fhir.jpa.model.sched.HapiJob; 024import ca.uhn.fhir.jpa.model.sched.IHasScheduledJobs; 025import ca.uhn.fhir.jpa.model.sched.ISchedulerService; 026import ca.uhn.fhir.jpa.model.sched.ScheduledJobDefinition; 027import org.quartz.JobExecutionContext; 028import org.springframework.beans.factory.annotation.Autowired; 029 030/** 031 * This service is responsible for scheduling a job that will submit messages 032 * to the subscription processing pipeline at a given interval. 033 */ 034public class AsyncResourceModifiedProcessingSchedulerSvc implements IHasScheduledJobs { 035 036 public static final long DEFAULT_SUBMISSION_INTERVAL_IN_MS = 5000; 037 038 public long mySubmissionIntervalInMilliSeconds; 039 040 public AsyncResourceModifiedProcessingSchedulerSvc() { 041 this(DEFAULT_SUBMISSION_INTERVAL_IN_MS); 042 } 043 044 public AsyncResourceModifiedProcessingSchedulerSvc(long theSubmissionIntervalInMilliSeconds) { 045 mySubmissionIntervalInMilliSeconds = theSubmissionIntervalInMilliSeconds; 046 } 047 048 @Override 049 public void scheduleJobs(ISchedulerService theSchedulerService) { 050 ScheduledJobDefinition jobDetail = new ScheduledJobDefinition(); 051 jobDetail.setId(getClass().getName()); 052 jobDetail.setJobClass(AsyncResourceModifiedProcessingSchedulerSvc.Job.class); 053 054 theSchedulerService.scheduleClusteredJob(mySubmissionIntervalInMilliSeconds, jobDetail); 055 } 056 057 public static class Job implements HapiJob { 058 @Autowired 059 private AsyncResourceModifiedSubmitterSvc myAsyncResourceModifiedSubmitterSvc; 060 061 @Override 062 public void execute(JobExecutionContext theContext) { 063 myAsyncResourceModifiedSubmitterSvc.runDeliveryPass(); 064 } 065 } 066}