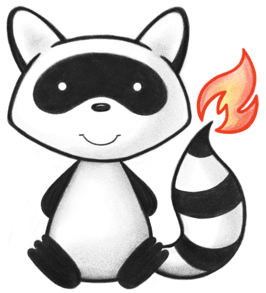
001/*- 002 * #%L 003 * HAPI FHIR Subscription Server 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.subscription.match.config; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.interceptor.api.IInterceptorBroadcaster; 024import ca.uhn.fhir.jpa.searchparam.matcher.SearchParamMatcher; 025import ca.uhn.fhir.jpa.subscription.channel.api.IChannelFactory; 026import ca.uhn.fhir.jpa.subscription.channel.subscription.SubscriptionChannelRegistry; 027import ca.uhn.fhir.jpa.subscription.channel.subscription.SubscriptionDeliveryChannelNamer; 028import ca.uhn.fhir.jpa.subscription.channel.subscription.SubscriptionDeliveryHandlerFactory; 029import ca.uhn.fhir.jpa.subscription.match.deliver.email.IEmailSender; 030import ca.uhn.fhir.jpa.subscription.match.deliver.email.SubscriptionDeliveringEmailSubscriber; 031import ca.uhn.fhir.jpa.subscription.match.deliver.message.SubscriptionDeliveringMessageSubscriber; 032import ca.uhn.fhir.jpa.subscription.match.deliver.resthook.SubscriptionDeliveringRestHookSubscriber; 033import ca.uhn.fhir.jpa.subscription.match.matcher.matching.CompositeInMemoryDaoSubscriptionMatcher; 034import ca.uhn.fhir.jpa.subscription.match.matcher.matching.DaoSubscriptionMatcher; 035import ca.uhn.fhir.jpa.subscription.match.matcher.matching.ISubscriptionMatcher; 036import ca.uhn.fhir.jpa.subscription.match.matcher.matching.InMemorySubscriptionMatcher; 037import ca.uhn.fhir.jpa.subscription.match.matcher.subscriber.MatchingQueueSubscriberLoader; 038import ca.uhn.fhir.jpa.subscription.match.matcher.subscriber.SubscriptionActivatingSubscriber; 039import ca.uhn.fhir.jpa.subscription.match.matcher.subscriber.SubscriptionMatchDeliverer; 040import ca.uhn.fhir.jpa.subscription.match.matcher.subscriber.SubscriptionMatchingSubscriber; 041import ca.uhn.fhir.jpa.subscription.match.matcher.subscriber.SubscriptionRegisteringSubscriber; 042import ca.uhn.fhir.jpa.subscription.match.registry.SubscriptionLoader; 043import ca.uhn.fhir.jpa.subscription.match.registry.SubscriptionRegistry; 044import ca.uhn.fhir.jpa.subscription.model.config.SubscriptionModelConfig; 045import ca.uhn.fhir.jpa.topic.SubscriptionTopicDispatcher; 046import ca.uhn.fhir.jpa.topic.SubscriptionTopicPayloadBuilder; 047import ca.uhn.fhir.jpa.topic.filter.InMemoryTopicFilterMatcher; 048import org.springframework.context.ApplicationContext; 049import org.springframework.context.annotation.Bean; 050import org.springframework.context.annotation.Import; 051import org.springframework.context.annotation.Lazy; 052import org.springframework.context.annotation.Primary; 053import org.springframework.context.annotation.Scope; 054 055/** 056 * This Spring config should be imported by a system that pulls messages off of the 057 * matching queue for processing, and handles delivery 058 */ 059@Import(SubscriptionModelConfig.class) 060public class SubscriptionProcessorConfig { 061 062 @Bean 063 public SubscriptionMatchingSubscriber subscriptionMatchingSubscriber() { 064 return new SubscriptionMatchingSubscriber(); 065 } 066 067 @Bean 068 public SubscriptionActivatingSubscriber subscriptionActivatingSubscriber() { 069 return new SubscriptionActivatingSubscriber(); 070 } 071 072 @Bean 073 public MatchingQueueSubscriberLoader subscriptionMatchingSubscriberLoader() { 074 return new MatchingQueueSubscriberLoader(); 075 } 076 077 @Bean 078 public SubscriptionRegisteringSubscriber subscriptionRegisteringSubscriber() { 079 return new SubscriptionRegisteringSubscriber(); 080 } 081 082 @Bean 083 public SubscriptionRegistry subscriptionRegistry() { 084 return new SubscriptionRegistry(); 085 } 086 087 @Bean 088 public SubscriptionDeliveryChannelNamer subscriptionDeliveryChannelNamer() { 089 return new SubscriptionDeliveryChannelNamer(); 090 } 091 092 @Bean 093 public SubscriptionLoader subscriptionLoader() { 094 return new SubscriptionLoader(); 095 } 096 097 @Bean 098 public SubscriptionChannelRegistry subscriptionChannelRegistry() { 099 return new SubscriptionChannelRegistry(); 100 } 101 102 @Bean 103 public SubscriptionDeliveryHandlerFactory subscriptionDeliveryHandlerFactory( 104 ApplicationContext theApplicationContext, IEmailSender theEmailSender) { 105 return new SubscriptionDeliveryHandlerFactory(theApplicationContext, theEmailSender); 106 } 107 108 @Bean 109 public SubscriptionMatchDeliverer subscriptionMatchDeliverer( 110 FhirContext theFhirContext, 111 IInterceptorBroadcaster theInterceptorBroadcaster, 112 SubscriptionChannelRegistry theSubscriptionChannelRegistry) { 113 return new SubscriptionMatchDeliverer( 114 theFhirContext, theInterceptorBroadcaster, theSubscriptionChannelRegistry); 115 } 116 117 @Bean 118 @Scope("prototype") 119 public SubscriptionDeliveringRestHookSubscriber subscriptionDeliveringRestHookSubscriber() { 120 return new SubscriptionDeliveringRestHookSubscriber(); 121 } 122 123 @Bean 124 @Scope("prototype") 125 public SubscriptionDeliveringMessageSubscriber subscriptionDeliveringMessageSubscriber( 126 IChannelFactory theChannelFactory) { 127 return new SubscriptionDeliveringMessageSubscriber(theChannelFactory); 128 } 129 130 @Bean 131 @Scope("prototype") 132 public SubscriptionDeliveringEmailSubscriber subscriptionDeliveringEmailSubscriber(IEmailSender theEmailSender) { 133 return new SubscriptionDeliveringEmailSubscriber(theEmailSender); 134 } 135 136 @Bean 137 public InMemorySubscriptionMatcher inMemorySubscriptionMatcher() { 138 return new InMemorySubscriptionMatcher(); 139 } 140 141 @Bean 142 public DaoSubscriptionMatcher daoSubscriptionMatcher() { 143 return new DaoSubscriptionMatcher(); 144 } 145 146 @Bean 147 @Primary 148 public ISubscriptionMatcher subscriptionMatcher( 149 DaoSubscriptionMatcher theDaoSubscriptionMatcher, 150 InMemorySubscriptionMatcher theInMemorySubscriptionMatcher) { 151 return new CompositeInMemoryDaoSubscriptionMatcher(theDaoSubscriptionMatcher, theInMemorySubscriptionMatcher); 152 } 153 154 @Lazy 155 @Bean 156 SubscriptionTopicPayloadBuilder subscriptionTopicPayloadBuilder(FhirContext theFhirContext) { 157 switch (theFhirContext.getVersion().getVersion()) { 158 case R4: 159 case R4B: 160 case R5: 161 return new SubscriptionTopicPayloadBuilder(theFhirContext); 162 default: 163 return null; 164 } 165 } 166 167 @Lazy 168 @Bean 169 SubscriptionTopicDispatcher subscriptionTopicDispatcher( 170 FhirContext theFhirContext, 171 SubscriptionRegistry theSubscriptionRegistry, 172 SubscriptionMatchDeliverer theSubscriptionMatchDeliverer, 173 SubscriptionTopicPayloadBuilder theSubscriptionTopicPayloadBuilder) { 174 return new SubscriptionTopicDispatcher( 175 theFhirContext, 176 theSubscriptionRegistry, 177 theSubscriptionMatchDeliverer, 178 theSubscriptionTopicPayloadBuilder); 179 } 180 181 @Bean 182 InMemoryTopicFilterMatcher inMemoryTopicFilterMatcher(SearchParamMatcher theSearchParamMatcher) { 183 return new InMemoryTopicFilterMatcher(theSearchParamMatcher); 184 } 185}