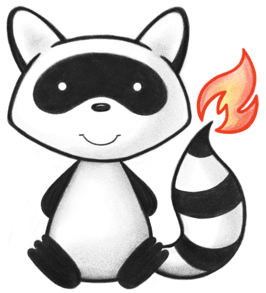
001/*- 002 * #%L 003 * HAPI FHIR Subscription Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.subscription.match.deliver.email; 021 022import ca.uhn.fhir.rest.server.mail.IMailSvc; 023import ca.uhn.fhir.util.StopWatch; 024import jakarta.annotation.Nonnull; 025import org.apache.commons.lang3.Validate; 026import org.simplejavamail.api.email.Email; 027import org.slf4j.Logger; 028import org.slf4j.LoggerFactory; 029 030public class EmailSenderImpl implements IEmailSender { 031 032 private static final Logger ourLog = LoggerFactory.getLogger(EmailSenderImpl.class); 033 034 private final IMailSvc myMailSvc; 035 036 public EmailSenderImpl(@Nonnull IMailSvc theMailSvc) { 037 Validate.notNull(theMailSvc); 038 myMailSvc = theMailSvc; 039 } 040 041 @Override 042 public void send(EmailDetails theDetails) { 043 StopWatch stopWatch = new StopWatch(); 044 045 ourLog.info( 046 "Sending email for subscription {} from [{}] to recipients: [{}]", 047 theDetails.getSubscriptionId(), 048 theDetails.getFrom(), 049 theDetails.getTo()); 050 051 Email email = theDetails.toEmail(); 052 053 myMailSvc.sendMail( 054 email, 055 () -> ourLog.info( 056 "Done sending email for subscription {} from [{}] to recipients: [{}] (took {}ms)", 057 theDetails.getSubscriptionId(), 058 theDetails.getFrom(), 059 theDetails.getTo(), 060 stopWatch.getMillis()), 061 (e) -> { 062 ourLog.error( 063 "Error sending email for subscription {} from [{}] to recipients: [{}] (took {}ms)", 064 theDetails.getSubscriptionId(), 065 theDetails.getFrom(), 066 theDetails.getTo(), 067 stopWatch.getMillis()); 068 ourLog.error("Error sending email", e); 069 }); 070 } 071}