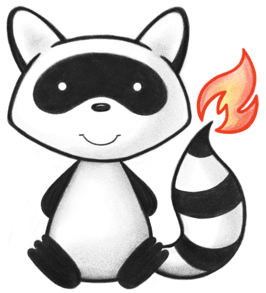
001/*- 002 * #%L 003 * HAPI FHIR Subscription Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.subscription.match.matcher.matching; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.context.RuntimeResourceDefinition; 024import ca.uhn.fhir.jpa.api.dao.DaoRegistry; 025import ca.uhn.fhir.jpa.api.dao.IFhirResourceDao; 026import ca.uhn.fhir.jpa.searchparam.MatchUrlService; 027import ca.uhn.fhir.jpa.searchparam.SearchParameterMap; 028import ca.uhn.fhir.jpa.searchparam.matcher.InMemoryMatchResult; 029import ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription; 030import ca.uhn.fhir.jpa.subscription.model.ResourceModifiedMessage; 031import ca.uhn.fhir.jpa.subscription.util.SubscriptionUtil; 032import ca.uhn.fhir.rest.api.server.IBundleProvider; 033import org.hl7.fhir.instance.model.api.IBaseResource; 034import org.hl7.fhir.instance.model.api.IIdType; 035import org.slf4j.Logger; 036import org.slf4j.LoggerFactory; 037import org.springframework.beans.factory.annotation.Autowired; 038 039public class DaoSubscriptionMatcher implements ISubscriptionMatcher { 040 private final Logger ourLog = LoggerFactory.getLogger(DaoSubscriptionMatcher.class); 041 042 @Autowired 043 DaoRegistry myDaoRegistry; 044 045 @Autowired 046 MatchUrlService myMatchUrlService; 047 048 @Autowired 049 private FhirContext myCtx; 050 051 @Override 052 public InMemoryMatchResult match(CanonicalSubscription theSubscription, ResourceModifiedMessage theMsg) { 053 IIdType id = theMsg.getPayloadId(myCtx); 054 String criteria = theSubscription.getCriteriaString(); 055 056 // Run the subscriptions query and look for matches, add the id as part of the criteria to avoid getting matches 057 // of previous resources rather than the recent resource 058 criteria += "&_id=" + id.toUnqualifiedVersionless().getValue(); 059 060 IBundleProvider results = performSearch(criteria, theSubscription); 061 062 ourLog.debug("Subscription check found {} results for query: {}", results.size(), criteria); 063 064 return InMemoryMatchResult.fromBoolean(results.size() > 0); 065 } 066 067 /** 068 * Search based on a query criteria 069 */ 070 private IBundleProvider performSearch(String theCriteria, CanonicalSubscription theSubscription) { 071 IFhirResourceDao<?> subscriptionDao = myDaoRegistry.getSubscriptionDao(); 072 RuntimeResourceDefinition responseResourceDef = 073 subscriptionDao.validateCriteriaAndReturnResourceDefinition(theCriteria); 074 SearchParameterMap responseCriteriaUrl = myMatchUrlService.translateMatchUrl(theCriteria, responseResourceDef); 075 076 IFhirResourceDao<? extends IBaseResource> responseDao = 077 myDaoRegistry.getResourceDao(responseResourceDef.getImplementingClass()); 078 responseCriteriaUrl.setLoadSynchronousUpTo(1); 079 080 return responseDao.search( 081 responseCriteriaUrl, SubscriptionUtil.createRequestDetailForPartitionedRequest(theSubscription)); 082 } 083}