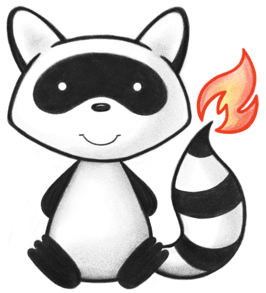
001/*- 002 * #%L 003 * HAPI FHIR Subscription Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.subscription.match.matcher.matching; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.i18n.Msg; 024import ca.uhn.fhir.jpa.searchparam.matcher.InMemoryMatchResult; 025import ca.uhn.fhir.jpa.searchparam.matcher.SearchParamMatcher; 026import ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription; 027import ca.uhn.fhir.jpa.subscription.model.ResourceModifiedMessage; 028import ca.uhn.fhir.rest.server.exceptions.InternalErrorException; 029import org.slf4j.Logger; 030import org.slf4j.LoggerFactory; 031import org.springframework.beans.factory.annotation.Autowired; 032 033public class InMemorySubscriptionMatcher implements ISubscriptionMatcher { 034 private static final Logger ourLog = LoggerFactory.getLogger(InMemorySubscriptionMatcher.class); 035 036 @Autowired 037 private FhirContext myContext; 038 039 @Autowired 040 private SearchParamMatcher mySearchParamMatcher; 041 042 @Override 043 public InMemoryMatchResult match(CanonicalSubscription theSubscription, ResourceModifiedMessage theMsg) { 044 try { 045 return mySearchParamMatcher.match( 046 theSubscription.getCriteriaString(), theMsg.getNewResource(myContext), null); 047 } catch (Exception e) { 048 ourLog.error("Failure in in-memory matcher", e); 049 throw new InternalErrorException( 050 Msg.code(1) + "Failure performing memory-match for resource ID[" + theMsg.getPayloadId(myContext) 051 + "] for subscription ID[" + theSubscription.getIdElementString() + "]: " + e.getMessage(), 052 e); 053 } 054 } 055}