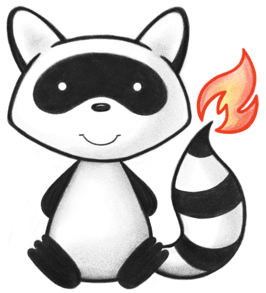
001/*- 002 * #%L 003 * HAPI FHIR Subscription Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.subscription.match.matcher.subscriber; 021 022import ca.uhn.fhir.IHapiBootOrder; 023import ca.uhn.fhir.context.FhirContext; 024import ca.uhn.fhir.jpa.model.config.SubscriptionSettings; 025import ca.uhn.fhir.jpa.subscription.channel.api.ChannelConsumerSettings; 026import ca.uhn.fhir.jpa.subscription.channel.api.IChannelReceiver; 027import ca.uhn.fhir.jpa.subscription.channel.subscription.SubscriptionChannelFactory; 028import ca.uhn.fhir.jpa.topic.SubscriptionTopicMatchingSubscriber; 029import ca.uhn.fhir.jpa.topic.SubscriptionTopicRegisteringSubscriber; 030import jakarta.annotation.PreDestroy; 031import org.slf4j.Logger; 032import org.slf4j.LoggerFactory; 033import org.springframework.beans.factory.annotation.Autowired; 034import org.springframework.context.event.ContextRefreshedEvent; 035import org.springframework.context.event.EventListener; 036import org.springframework.core.annotation.Order; 037 038import static ca.uhn.fhir.jpa.subscription.match.matcher.subscriber.SubscriptionMatchingSubscriber.SUBSCRIPTION_MATCHING_CHANNEL_NAME; 039 040public class MatchingQueueSubscriberLoader { 041 protected IChannelReceiver myMatchingChannel; 042 private static final Logger ourLog = LoggerFactory.getLogger(MatchingQueueSubscriberLoader.class); 043 044 @Autowired 045 FhirContext myFhirContext; 046 047 @Autowired 048 private SubscriptionMatchingSubscriber mySubscriptionMatchingSubscriber; 049 050 @Autowired(required = false) 051 private SubscriptionTopicMatchingSubscriber mySubscriptionTopicMatchingSubscriber; 052 053 @Autowired 054 private SubscriptionChannelFactory mySubscriptionChannelFactory; 055 056 @Autowired 057 private SubscriptionRegisteringSubscriber mySubscriptionRegisteringSubscriber; 058 059 @Autowired(required = false) 060 private SubscriptionTopicRegisteringSubscriber mySubscriptionTopicRegisteringSubscriber; 061 062 @Autowired 063 private SubscriptionActivatingSubscriber mySubscriptionActivatingSubscriber; 064 065 @Autowired 066 private SubscriptionSettings mySubscriptionSettings; 067 068 @EventListener(ContextRefreshedEvent.class) 069 @Order(IHapiBootOrder.SUBSCRIPTION_MATCHING_CHANNEL_HANDLER) 070 public void subscribeToMatchingChannel() { 071 if (myMatchingChannel == null) { 072 myMatchingChannel = mySubscriptionChannelFactory.newMatchingReceivingChannel( 073 SUBSCRIPTION_MATCHING_CHANNEL_NAME, getChannelConsumerSettings()); 074 } 075 if (myMatchingChannel != null) { 076 myMatchingChannel.subscribe(mySubscriptionMatchingSubscriber); 077 myMatchingChannel.subscribe(mySubscriptionActivatingSubscriber); 078 myMatchingChannel.subscribe(mySubscriptionRegisteringSubscriber); 079 ourLog.info( 080 "Subscription Matching Subscriber subscribed to Matching Channel {} with name {}", 081 myMatchingChannel.getClass().getName(), 082 SUBSCRIPTION_MATCHING_CHANNEL_NAME); 083 if (mySubscriptionTopicMatchingSubscriber != null) { 084 ourLog.info("Starting SubscriptionTopic Matching Subscriber"); 085 myMatchingChannel.subscribe(mySubscriptionTopicMatchingSubscriber); 086 } 087 if (mySubscriptionTopicRegisteringSubscriber != null) { 088 myMatchingChannel.subscribe(mySubscriptionTopicRegisteringSubscriber); 089 } 090 } 091 } 092 093 private ChannelConsumerSettings getChannelConsumerSettings() { 094 ChannelConsumerSettings channelConsumerSettings = new ChannelConsumerSettings(); 095 channelConsumerSettings.setQualifyChannelName( 096 mySubscriptionSettings.isQualifySubscriptionMatchingChannelName()); 097 return channelConsumerSettings; 098 } 099 100 @SuppressWarnings("unused") 101 @PreDestroy 102 public void stop() throws Exception { 103 if (myMatchingChannel != null) { 104 ourLog.info( 105 "Destroying matching Channel {} with name {}", 106 myMatchingChannel.getClass().getName(), 107 SUBSCRIPTION_MATCHING_CHANNEL_NAME); 108 myMatchingChannel.destroy(); 109 myMatchingChannel.unsubscribe(mySubscriptionMatchingSubscriber); 110 myMatchingChannel.unsubscribe(mySubscriptionActivatingSubscriber); 111 myMatchingChannel.unsubscribe(mySubscriptionRegisteringSubscriber); 112 } 113 } 114}