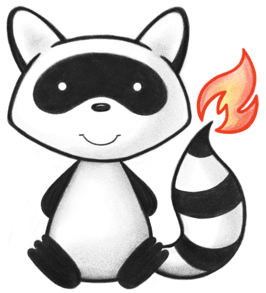
001/*- 002 * #%L 003 * HAPI FHIR Subscription Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.subscription.match.matcher.subscriber; 021 022import ca.uhn.fhir.interceptor.model.RequestPartitionId; 023import ca.uhn.fhir.jpa.subscription.match.registry.ActiveSubscription; 024import ca.uhn.fhir.jpa.subscription.model.CanonicalSubscription; 025import ca.uhn.fhir.jpa.subscription.model.ResourceModifiedMessage; 026import ca.uhn.fhir.jpa.topic.SubscriptionTopicDispatchRequest; 027import ca.uhn.fhir.rest.api.RestOperationTypeEnum; 028import ca.uhn.fhir.rest.server.messaging.BaseResourceModifiedMessage; 029import jakarta.annotation.Nonnull; 030import org.hl7.fhir.instance.model.api.IBaseBundle; 031import org.hl7.fhir.instance.model.api.IBaseResource; 032import org.hl7.fhir.instance.model.api.IIdType; 033 034public class SubscriptionDeliveryRequest { 035 // One of these two will be populated 036 private final IBaseResource myPayload; 037 private final IIdType myPayloadId; 038 private final ActiveSubscription myActiveSubscription; 039 private final RestOperationTypeEnum myRestOperationType; 040 private final RequestPartitionId myRequestPartitionId; 041 private final String myTransactionId; 042 043 public SubscriptionDeliveryRequest( 044 IBaseBundle theBundlePayload, 045 ActiveSubscription theActiveSubscription, 046 SubscriptionTopicDispatchRequest theSubscriptionTopicDispatchRequest) { 047 myPayload = theBundlePayload; 048 myPayloadId = null; 049 myActiveSubscription = theActiveSubscription; 050 myRestOperationType = theSubscriptionTopicDispatchRequest.getRequestType(); 051 myRequestPartitionId = theSubscriptionTopicDispatchRequest.getRequestPartitionId(); 052 myTransactionId = theSubscriptionTopicDispatchRequest.getTransactionId(); 053 } 054 055 public SubscriptionDeliveryRequest( 056 @Nonnull IBaseResource thePayload, 057 @Nonnull ResourceModifiedMessage theMsg, 058 @Nonnull ActiveSubscription theActiveSubscription) { 059 myPayload = thePayload; 060 myPayloadId = null; 061 myActiveSubscription = theActiveSubscription; 062 myRestOperationType = theMsg.getOperationType().asRestOperationType(); 063 myRequestPartitionId = theMsg.getPartitionId(); 064 myTransactionId = theMsg.getTransactionId(); 065 } 066 067 public SubscriptionDeliveryRequest( 068 @Nonnull IIdType thePayloadId, 069 @Nonnull ResourceModifiedMessage theMsg, 070 @Nonnull ActiveSubscription theActiveSubscription) { 071 myPayload = null; 072 myPayloadId = thePayloadId; 073 myActiveSubscription = theActiveSubscription; 074 myRestOperationType = theMsg.getOperationType().asRestOperationType(); 075 myRequestPartitionId = theMsg.getPartitionId(); 076 myTransactionId = theMsg.getTransactionId(); 077 } 078 079 public IBaseResource getPayload() { 080 return myPayload; 081 } 082 083 public ActiveSubscription getActiveSubscription() { 084 return myActiveSubscription; 085 } 086 087 public RestOperationTypeEnum getRestOperationType() { 088 return myRestOperationType; 089 } 090 091 public BaseResourceModifiedMessage.OperationTypeEnum getOperationType() { 092 return BaseResourceModifiedMessage.OperationTypeEnum.from(myRestOperationType); 093 } 094 095 public RequestPartitionId getRequestPartitionId() { 096 return myRequestPartitionId; 097 } 098 099 public String getTransactionId() { 100 return myTransactionId; 101 } 102 103 public CanonicalSubscription getSubscription() { 104 return myActiveSubscription.getSubscription(); 105 } 106 107 public IIdType getPayloadId() { 108 return myPayloadId; 109 } 110 111 public boolean hasPayload() { 112 return myPayload != null; 113 } 114}