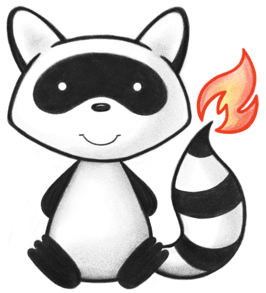
001/*- 002 * #%L 003 * HAPI FHIR Subscription Server 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.subscription.submit.interceptor; 021 022import ca.uhn.fhir.interceptor.api.IInterceptorService; 023import ca.uhn.fhir.jpa.model.config.SubscriptionSettings; 024import ca.uhn.fhir.jpa.topic.SubscriptionTopicValidatingInterceptor; 025import com.google.common.annotations.VisibleForTesting; 026import jakarta.annotation.Nonnull; 027import jakarta.annotation.Nullable; 028import jakarta.annotation.PostConstruct; 029import org.hl7.fhir.dstu2.model.Subscription; 030import org.slf4j.Logger; 031import org.slf4j.LoggerFactory; 032 033import java.util.Set; 034 035public class SubscriptionSubmitInterceptorLoader { 036 private static final Logger ourLog = LoggerFactory.getLogger(SubscriptionSubmitInterceptorLoader.class); 037 038 @Nonnull 039 private IInterceptorService myInterceptorService; 040 041 @Nonnull 042 private final SubscriptionSettings mySubscriptionSettings; 043 044 @Nonnull 045 private final SubscriptionMatcherInterceptor mySubscriptionMatcherInterceptor; 046 047 @Nonnull 048 private final SubscriptionValidatingInterceptor mySubscriptionValidatingInterceptor; 049 050 @Nullable 051 private final SubscriptionTopicValidatingInterceptor mySubscriptionTopicValidatingInterceptor; 052 053 private boolean mySubscriptionValidatingInterceptorRegistered; 054 private boolean mySubscriptionMatcherInterceptorRegistered; 055 private boolean mySubscriptionTopicValidatingInterceptorRegistered; 056 057 public SubscriptionSubmitInterceptorLoader( 058 @Nonnull IInterceptorService theInterceptorService, 059 @Nonnull SubscriptionSettings theSubscriptionSettings, 060 @Nonnull SubscriptionMatcherInterceptor theSubscriptionMatcherInterceptor, 061 @Nonnull SubscriptionValidatingInterceptor theSubscriptionValidatingInterceptor, 062 @Nullable SubscriptionTopicValidatingInterceptor theSubscriptionTopicValidatingInterceptor) { 063 setInterceptorService(theInterceptorService); 064 mySubscriptionSettings = theSubscriptionSettings; 065 mySubscriptionMatcherInterceptor = theSubscriptionMatcherInterceptor; 066 mySubscriptionValidatingInterceptor = theSubscriptionValidatingInterceptor; 067 mySubscriptionTopicValidatingInterceptor = theSubscriptionTopicValidatingInterceptor; 068 } 069 070 @PostConstruct 071 public void start() { 072 Set<Subscription.SubscriptionChannelType> supportedSubscriptionTypes = 073 mySubscriptionSettings.getSupportedSubscriptionTypes(); 074 075 if (supportedSubscriptionTypes.isEmpty()) { 076 ourLog.info( 077 "Subscriptions are disabled on this server. Subscriptions will not be activated and incoming resources will not be matched against subscriptions."); 078 } else { 079 if (!mySubscriptionMatcherInterceptorRegistered) { 080 ourLog.info("Registering subscription matcher interceptor"); 081 myInterceptorService.registerInterceptor(mySubscriptionMatcherInterceptor); 082 mySubscriptionMatcherInterceptorRegistered = true; 083 } 084 } 085 086 if (!mySubscriptionValidatingInterceptorRegistered) { 087 myInterceptorService.registerInterceptor(mySubscriptionValidatingInterceptor); 088 mySubscriptionValidatingInterceptorRegistered = true; 089 } 090 091 if (mySubscriptionTopicValidatingInterceptor != null && !mySubscriptionTopicValidatingInterceptorRegistered) { 092 myInterceptorService.registerInterceptor(mySubscriptionTopicValidatingInterceptor); 093 mySubscriptionTopicValidatingInterceptorRegistered = true; 094 } 095 } 096 097 protected void setInterceptorService(IInterceptorService theInterceptorService) { 098 myInterceptorService = theInterceptorService; 099 } 100 101 protected IInterceptorService getInterceptorService() { 102 return myInterceptorService; 103 } 104 105 @VisibleForTesting 106 public void unregisterInterceptorsForUnitTest() { 107 myInterceptorService.unregisterInterceptor(mySubscriptionMatcherInterceptor); 108 myInterceptorService.unregisterInterceptor(mySubscriptionValidatingInterceptor); 109 mySubscriptionValidatingInterceptorRegistered = false; 110 mySubscriptionMatcherInterceptorRegistered = false; 111 } 112}