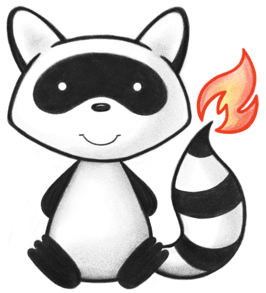
001/*- 002 * #%L 003 * HAPI FHIR Subscription Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.topic; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.jpa.api.dao.DaoRegistry; 024import ca.uhn.fhir.jpa.searchparam.matcher.SearchParamMatcher; 025import ca.uhn.fhir.jpa.subscription.config.SubscriptionConfig; 026import ca.uhn.fhir.jpa.subscription.submit.interceptor.validator.SubscriptionQueryValidator; 027import ca.uhn.fhir.jpa.util.MemoryCacheService; 028import ca.uhn.fhir.rest.server.util.ISearchParamRegistry; 029import ca.uhn.hapi.converters.canonical.VersionCanonicalizer; 030import org.springframework.context.annotation.Bean; 031import org.springframework.context.annotation.Configuration; 032import org.springframework.context.annotation.Import; 033import org.springframework.context.annotation.Lazy; 034 035@Configuration 036@Import(SubscriptionConfig.class) 037public class SubscriptionTopicConfig { 038 @Bean 039 public SubscriptionTopicMatchingListener subscriptionTopicMatchingSubscriber( 040 FhirContext theFhirContext, MemoryCacheService memoryCacheService) { 041 switch (theFhirContext.getVersion().getVersion()) { 042 case R4: 043 case R5: 044 case R4B: 045 return new SubscriptionTopicMatchingListener(theFhirContext, memoryCacheService); 046 default: 047 return null; 048 } 049 } 050 051 @Bean 052 @Lazy 053 public SubscriptionTopicRegistry subscriptionTopicRegistry() { 054 return new SubscriptionTopicRegistry(); 055 } 056 057 @Bean 058 @Lazy 059 public SubscriptionTopicSupport subscriptionTopicSupport( 060 FhirContext theFhirContext, DaoRegistry theDaoRegistry, SearchParamMatcher theSearchParamMatcher) { 061 return new SubscriptionTopicSupport(theFhirContext, theDaoRegistry, theSearchParamMatcher); 062 } 063 064 @Bean 065 public ISubscriptionTopicLoader subscriptionTopicLoader( 066 FhirContext theFhirContext, 067 SubscriptionTopicRegistry theSubscriptionTopicRegistry, 068 ISearchParamRegistry theSearchParamRegistry) { 069 VersionCanonicalizer versionCanonicalizer = new VersionCanonicalizer(theFhirContext); 070 switch (theFhirContext.getVersion().getVersion()) { 071 case R4: 072 return new R4SubscriptionTopicLoader( 073 versionCanonicalizer, theSubscriptionTopicRegistry, theSearchParamRegistry); 074 case R5: 075 case R4B: 076 return new SubscriptionTopicLoader( 077 versionCanonicalizer, theSubscriptionTopicRegistry, theSearchParamRegistry); 078 default: 079 return null; 080 } 081 } 082 083 @Bean 084 public SubscriptionTopicRegisteringListener subscriptionTopicRegisteringSubscriber(FhirContext theFhirContext) { 085 switch (theFhirContext.getVersion().getVersion()) { 086 case R5: 087 case R4B: 088 return new SubscriptionTopicRegisteringListener(); 089 default: 090 return null; 091 } 092 } 093 094 @Bean 095 public SubscriptionTopicValidatingInterceptor subscriptionTopicValidatingInterceptor( 096 FhirContext theFhirContext, SubscriptionQueryValidator theSubscriptionQueryValidator) { 097 switch (theFhirContext.getVersion().getVersion()) { 098 case R5: 099 case R4B: 100 return new SubscriptionTopicValidatingInterceptor(theFhirContext, theSubscriptionQueryValidator); 101 default: 102 return null; 103 } 104 } 105}